Linear algebra is a cornerstone of many superior mathematical ideas and is extensively utilized in knowledge science, machine studying, laptop imaginative and prescient, and engineering. One of many basic ideas in linear algebra is eigenvectors, usually paired with eigenvalues. However what precisely is an eigenvector, and why is it so vital?
This text breaks down the idea of eigenvectors in a easy and intuitive method, making it straightforward for anybody to know.
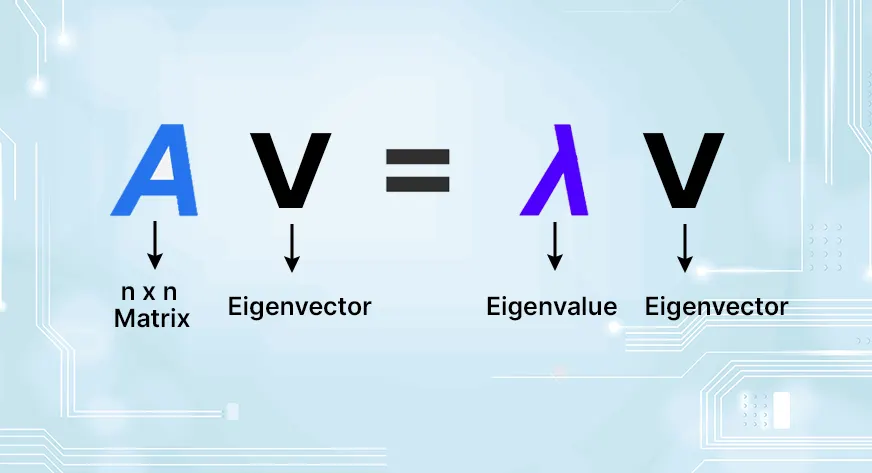
What’s an Eigenvector?
A sq. matrix is associates with a particular sort of vector known as an eigenvector. When the matrix acts on the eigenvector, it retains the path of the eigenvector unchanged and solely scales it by a scalar worth known as the eigenvalue.
In mathematical phrases, for a sq. matrix A, a non-zero vector v is an eigenvector if:
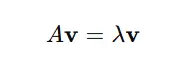
Right here:
- A is the matrix.
- v is the eigenvector.
- λ is the eigenvalue (a scalar).
Instinct Behind Eigenvectors
Think about you have got a matrix A representing a linear transformation, comparable to stretching, rotating, or scaling a 2D area. When this transformation is utilized to a vector v:
- Most vectors will change their path and magnitude.
- Some particular vectors, nevertheless, will solely be scaled however not rotated or flipped. These particular vectors are eigenvectors.
For instance:
- If λ>1, the eigenvector is stretched.
- If 0<λ<1, the eigenvector is compressed.
- If λ=−1, the eigenvector flips its path however maintains the identical size.
Why Are Eigenvectors Essential?
Eigenvectors play an important function in varied mathematical and real-world functions:
- Principal Element Evaluation (PCA): PCA is a broadly used approach for dimensionality discount. Eigenvectors are used to find out the principal elements of the information, which seize the utmost variance and assist establish a very powerful options.
- Google PageRank: The algorithm that ranks net pages makes use of eigenvectors of a matrix representing the hyperlinks between net pages. The principal eigenvector helps decide the relative significance of every web page.
- Quantum Mechanics: In physics, eigenvectors and eigenvalues describe the states of a system and their measurable properties, comparable to power ranges.
- Pc Imaginative and prescient: Eigenvectors are utilized in facial recognition methods, significantly in methods like Eigenfaces, the place they assist symbolize photos as linear mixtures of great options.
- Vibrational Evaluation: In engineering, eigenvectors describe the modes of vibration in constructions like bridges and buildings.
Methods to Compute Eigenvectors?
To seek out eigenvectors, observe these steps:
- Arrange the eigenvalue equation: Begin with Av=λv and rewrite it as (A−λI)v=0, the place I is the identification matrix. Remedy for eigenvalues: Discover eigenvectors:
- Remedy for eigenvalues: Compute det(A−λI)=0 to search out the eigenvalues λ.
- Discover eigenvectors: Substitute every eigenvalue λ into (A−λI)v=0 and remedy for v.
Instance: Eigenvectors in Motion
Take into account a matrix:
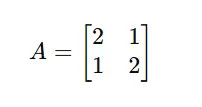
Step 1: Discover eigenvalues λ.
Remedy det(A−λI)=0:
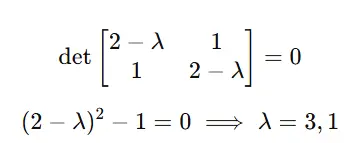
Step 2: Discover eigenvectors for every λ.
For λ=3:
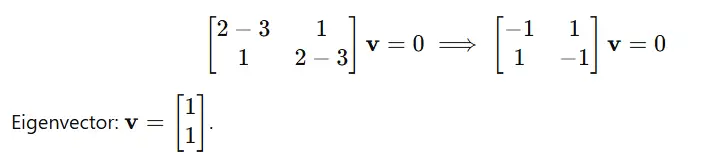
For λ=1:
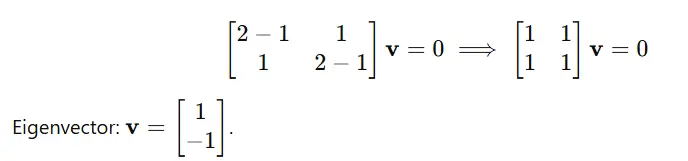
Python Implementation
Let’s compute the eigenvalues and eigenvectors of a matrix utilizing Python.
Instance Matrix
Take into account the matrix:
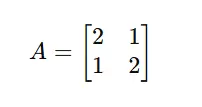
Code Implementation
import numpy as np
# Outline the matrix
A = np.array([[2, 1], [1, 2]])
# Compute eigenvalues and eigenvectors
eigenvalues, eigenvectors = np.linalg.eig(A)
# Show outcomes
print("Matrix A:")
print(A)
print("nEigenvalues:")
print(eigenvalues)
print("nEigenvectors:")
print(eigenvectors)
Output:
Matrix A:
[[2 1]
[1 2]]
Eigenvalues:
[3. 1.]
Eigenvectors:
[[ 0.70710678 -0.70710678]
[ 0.70710678 0.70710678]]
Visualizing Eigenvectors
You may visualize how eigenvectors behave below the transformation outlined by matrix A.
Visualization Code
import matplotlib.pyplot as plt
# Outline eigenvectors
eig_vec1 = eigenvectors[:, 0]
eig_vec2 = eigenvectors[:, 1]
# Plot authentic eigenvectors
plt.quiver(0, 0, eig_vec1[0], eig_vec1[1], angles="xy", scale_units="xy", scale=1, colour="r", label="Eigenvector 1")
plt.quiver(0, 0, eig_vec2[0], eig_vec2[1], angles="xy", scale_units="xy", scale=1, colour="b", label="Eigenvector 2")
# Alter plot settings
plt.xlim(-1, 1)
plt.ylim(-1, 1)
plt.axhline(0, colour="grey", linewidth=0.5)
plt.axvline(0, colour="grey", linewidth=0.5)
plt.grid(colour="lightgray", linestyle="--", linewidth=0.5)
plt.legend()
plt.title("Eigenvectors of Matrix A")
plt.present()
This code will produce a plot exhibiting the eigenvectors of AAA, illustrating their instructions and the way they continue to be unchanged below the transformation.
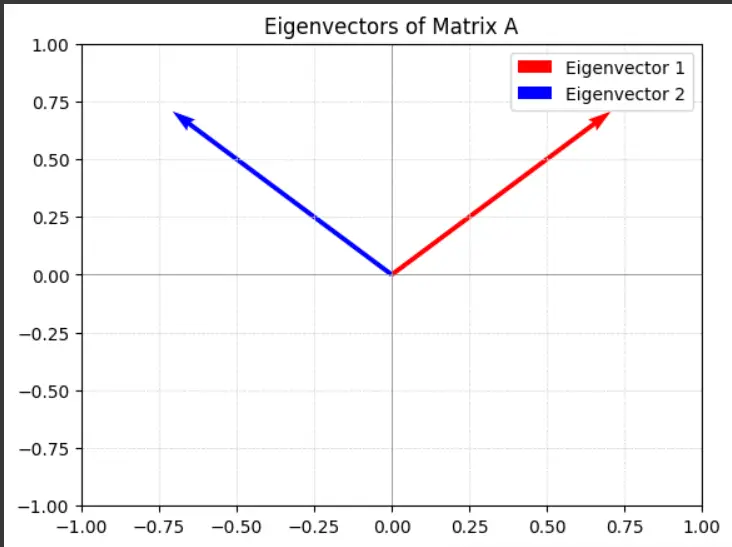
Key Takeaways
- Eigenvectors are particular vectors that stay in the identical path when reworked by a matrix.
- They’re paired with eigenvalues, which decide how a lot the eigenvectors are scaled.
- Eigenvectors have important functions in knowledge science, machine studying, engineering, and physics.
- Python offers instruments like NumPy to compute eigenvalues and eigenvectors simply.
Conclusion
Eigenvectors are a cornerstone idea in linear algebra, with far-reaching functions in knowledge science, engineering, physics, and past. They symbolize the essence of how a matrix transformation impacts sure particular instructions, making them indispensable in areas like dimensionality discount, picture processing, and vibrational evaluation.
By understanding and computing eigenvectors, you unlock a robust mathematical device that allows you to remedy advanced issues with readability and precision. With Python’s strong libraries like NumPy, exploring eigenvectors turns into simple, permitting you to visualise and apply these ideas in real-world eventualities.
Whether or not you’re constructing machine studying fashions, analyzing structural dynamics, or diving into quantum mechanics, a strong understanding of eigenvectors is a talent that can serve you effectively in your journey.
Continuously Requested Questions
Ans. Scalars that symbolize how a lot a change scales an eigenvector are known as eigenvalues. Vectors that stay in the identical path (although probably reversed or scaled) throughout a change are known as eigenvectors.
Ans. Not all matrices have eigenvectors. Solely sq. matrices can have eigenvectors, and even then, some matrices (e.g., faulty matrices) might not have an entire set of eigenvectors.
Ans. Eigenvectors will not be distinctive as a result of any scalar a number of of an eigenvector can also be an eigenvector. Nonetheless, their path stays constant for a given eigenvalue.
Ans. Eigenvectors are utilized in dimensionality discount methods like Principal Element Evaluation (PCA), the place they assist establish the principal elements of knowledge. This enables for decreasing the variety of options whereas preserving most variance.
Ans. If an eigenvalue is zero, it signifies that the transformation squashes the corresponding eigenvector into the zero vector. This usually pertains to the matrix being singular (non-invertible).