Evaluating massive language fashions (LLMs) is important. It’s essential to perceive how properly they carry out and guarantee they meet your requirements. The Hugging Face Consider library gives a useful set of instruments for this process. This information reveals you find out how to use the Consider library to evaluate LLMs with sensible code examples.
Understanding the Hugging Face Consider Library
The Hugging Face Consider library gives instruments for various analysis wants. These instruments fall into three primary classes:
- Metrics: These measure a mannequin’s efficiency by evaluating its predictions to floor reality labels. Examples embrace accuracy, F1-score, BLEU, and ROUGE.
- Comparisons: These assist evaluate two fashions, usually by inspecting how their predictions align with one another or with reference labels.
- Measurements: These instruments examine the properties of datasets themselves, like calculating textual content complexity or label distributions.
You’ll be able to entry all these analysis modules utilizing a single operate: consider.load().
Getting Began
Set up
First, you want to set up the library. Open your terminal or command immediate and run:
pip set up consider
pip set up rouge_score # Wanted for textual content technology metrics
pip set up consider[visualization] # For plotting capabilities
These instructions set up the core consider library, the rouge_score package deal (required for the ROUGE metric usually utilized in summarization), and optionally available dependencies for visualization like radar plots.
Loading an Analysis Module
To make use of a selected analysis instrument, you load it by identify. For example, to load the accuracy metric:
import consider
accuracy_metric = consider.load("accuracy")
print("Accuracy metric loaded.")
Output:

This code imports the consider library and masses the accuracy metric object. You’ll use this object to compute accuracy scores.
Primary Analysis Examples
Let’s stroll by way of some widespread analysis situations.
Computing Accuracy Straight
You’ll be able to compute a metric by offering all references (floor reality) and predictions without delay.
import consider
# Load the accuracy metric
accuracy_metric = consider.load("accuracy")
# Pattern floor reality and predictions
references = [0, 1, 0, 1]
predictions = [1, 0, 0, 1]
# Compute accuracy
end result = accuracy_metric.compute(references=references, predictions=predictions)
print(f"Direct computation end result: {end result}")
# Instance with exact_match metric
exact_match_metric = consider.load('exact_match')
match_result = exact_match_metric.compute(references=['hello world'], predictions=['hello world'])
no_match_result = exact_match_metric.compute(references=['hello'], predictions=['hell'])
print(f"Actual match end result (match): {match_result}")
print(f"Actual match end result (no match): {no_match_result}")
Output:

Clarification:
- We outline two lists: references holds the right labels, and predictions holds the mannequin’s outputs.
- The compute technique takes these lists and calculates the accuracy, returning the end result as a dictionary.
- We additionally present the exact_match metric, which checks if the prediction completely matches the reference.
Incremental Analysis (Utilizing add_batch)
For giant datasets, processing predictions in batches may be extra memory-efficient. You’ll be able to add batches incrementally and compute the ultimate rating on the finish.
import consider
# Load the accuracy metric
accuracy_metric = consider.load("accuracy")
# Pattern batches of refrences and predictions
references_batch1 = [0, 1]
predictions_batch1 = [1, 0]
references_batch2 = [0, 1]
predictions_batch2 = [0, 1]
# Add batches incrementally
accuracy_metric.add_batch(references=references_batch1, predictions=predictions_batch1)
accuracy_metric.add_batch(references=references_batch2, predictions=predictions_batch2)
# Compute ultimate accuracy
final_result = accuracy_metric.compute()
print(f"Incremental computation end result: {final_result}")
Output:

Clarification:
- We simulate processing information in two batches.
- add_batch updates the metric’s inner state with every batch.
- Calling compute() with out arguments calculates the metric over all added batches.
Combining A number of Metrics
You usually wish to calculate a number of metrics concurrently (e.g., accuracy, F1, precision, recall for classification). The consider.mix operate simplifies this.
import consider
# Mix a number of classification metrics
clf_metrics = consider.mix(["accuracy", "f1", "precision", "recall"])
# Pattern information
predictions = [0, 1, 0]
references = [0, 1, 1] # Notice: The final prediction is wrong
# Compute all metrics without delay
outcomes = clf_metrics.compute(predictions=predictions, references=references)
print(f"Mixed metrics end result: {outcomes}")
Output:

Clarification:
- consider.mix takes a listing of metric names and returns a mixed analysis object.
- Calling compute on this object calculates all the required metrics utilizing the identical enter information.
Utilizing Measurements
Measurements can be utilized to investigate datasets. Right here’s find out how to use the word_length measurement:
import consider
# Load the word_length measurement
# Notice: Could require NLTK information obtain on first run
strive:
word_length = consider.load("word_length", module_type="measurement")
information = ["hello world", "this is another sentence"]
outcomes = word_length.compute(information=information)
print(f"Phrase size measurement end result: {outcomes}")
besides Exception as e:
print(f"Couldn't run word_length measurement, probably NLTK information lacking: {e}")
print("Making an attempt NLTK obtain...")
import nltk
nltk.obtain('punkt') # Uncomment and run if wanted
Output:

Clarification:
- We load word_length and specify module_type=”measurement”.
- The compute technique takes the dataset (a listing of strings right here) as enter.
- It returns statistics in regards to the phrase lengths within the supplied information. (Notice: Requires nltk and its ‘punkt’ tokenizer information).
Evaluating Particular NLP Duties
Completely different NLP duties require particular metrics. Hugging Face Consider contains many commonplace ones.
Machine Translation (BLEU)
BLEU (Bilingual Analysis Understudy) is widespread for translation high quality. It measures n-gram overlap between the mannequin’s translation (speculation) and reference translations.
import consider
def evaluate_machine_translation(hypotheses, references):
"""Calculates BLEU rating for machine translation."""
bleu_metric = consider.load("bleu")
outcomes = bleu_metric.compute(predictions=hypotheses, references=references)
# Extract the primary BLEU rating
bleu_score = outcomes["bleu"]
return bleu_score
# Instance hypotheses (mannequin translations)
hypotheses = ["the cat sat on mat.", "the dog played in garden."]
# Instance references (right translations, can have a number of per speculation)
references = [["the cat sat on the mat."], ["the dog played in the garden."]]
bleu_score = evaluate_machine_translation(hypotheses, references)
print(f"BLEU Rating: {bleu_score:.4f}") # Format for readability
Output:

Clarification:
- The operate masses the BLEU metric.
- It computes the rating evaluating predicted translations (hypotheses) towards a number of right references.
- The next BLEU rating (nearer to 1.0) usually signifies higher translation high quality, suggesting extra overlap with reference translations. A rating round 0.51 suggests average overlap.
Named Entity Recognition (NER – utilizing seqeval)
For sequence labeling duties like NER, metrics like precision, recall, and F1-score per entity kind are helpful. The seqeval metric handles this format (e.g., B-PER, I-PER, O tags).
To run the next code, seqeval library can be required. It could possibly be put in by working the next command:
pip set up seqeval
Code:
import consider
# Load the seqeval metric
strive:
seqeval_metric = consider.load("seqeval")
# Instance labels (utilizing IOB format)
true_labels = [['O', 'B-PER', 'I-PER', 'O'], ['B-LOC', 'I-LOC', 'O']]
predicted_labels = [['O', 'B-PER', 'I-PER', 'O'], ['B-LOC', 'I-LOC', 'O']] # Instance: Good prediction right here
outcomes = seqeval_metric.compute(predictions=predicted_labels, references=true_labels)
print("Seqeval Outcomes (per entity kind):")
# Print outcomes properly
for key, worth in outcomes.objects():
if isinstance(worth, dict):
print(f" {key}: Precision={worth['precision']:.2f}, Recall={worth['recall']:.2f}, F1={worth['f1']:.2f}, Quantity={worth['number']}")
else:
print(f" {key}: {worth:.4f}")
besides ModuleNotFoundError:
print("Seqeval metric not put in. Run: pip set up seqeval")
Output:
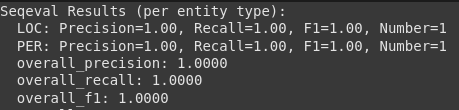
Clarification:
- We load the seqeval metric.
- It takes lists of lists, the place every inside listing represents the tags for a sentence.
- The compute technique returns detailed precision, recall, and F1 scores for every entity kind recognized (like PER for Particular person, LOC for Location) and total scores.
Textual content Summarization (ROUGE)
ROUGE (Recall-Oriented Understudy for Gisting Analysis) compares a generated abstract towards reference summaries, specializing in overlapping n-grams and longest widespread subsequences.
import consider
def simple_summarizer(textual content):
"""A really primary summarizer - simply takes the primary sentence."""
strive:
sentences = textual content.break up(".")
return sentences[0].strip() + "." if sentences[0].strip() else ""
besides:
return "" # Deal with empty or malformed textual content
# Load ROUGE metric
rouge_metric = consider.load("rouge")
# Instance textual content and reference abstract
textual content = "At this time is a wonderful day. The solar is shining and the birds are singing. I'm going for a stroll within the park."
reference = "The climate is nice in the present day."
# Generate abstract utilizing the straightforward operate
prediction = simple_summarizer(textual content)
print(f"Generated Abstract: {prediction}")
print(f"Reference Abstract: {reference}")
# Compute ROUGE scores
rouge_results = rouge_metric.compute(predictions=[prediction], references=[reference])
print(f"ROUGE Scores: {rouge_results}")
Output:
Generated Abstract: At this time is a wonderful day.Reference Abstract: The climate is nice in the present day.
ROUGE Scores: {'rouge1': np.float64(0.4000000000000001), 'rouge2':
np.float64(0.0), 'rougeL': np.float64(0.20000000000000004), 'rougeLsum':
np.float64(0.20000000000000004)}
Clarification:
- We load the rouge metric.
- We outline a simplistic summarizer for demonstration.
- compute calculates completely different ROUGE scores:
- Scores nearer to 1.0 point out increased similarity to the reference abstract. The low scores right here replicate the essential nature of our simple_summarizer.
Query Answering (SQuAD)
The SQuAD metric is used for extractive query answering benchmarks. It calculates Actual Match (EM) and F1-score.
import consider
# Load the SQuAD metric
squad_metric = consider.load("squad")
# Instance predictions and references format for SQuAD
predictions = [{'prediction_text': '1976', 'id': '56e10a3be3433e1400422b22'}]
references = [{'answers': {'answer_start': [97], 'textual content': ['1976']}, 'id': '56e10a3be3433e1400422b22'}]
outcomes = squad_metric.compute(predictions=predictions, references=references)
print(f"SQuAD Outcomes: {outcomes}")
Output:

Clarification:
- Hundreds the squad metric.
- Takes predictions and references in a selected dictionary format, together with the anticipated textual content and the bottom reality solutions with their begin positions.
- exact_match: Proportion of predictions that precisely match one of many floor reality solutions.
- f1: Common F1 rating over all questions, contemplating partial matches on the token stage.
Superior Analysis with the Evaluator Class
The Evaluator class streamlines the method by integrating mannequin loading, inference, and metric calculation. It’s significantly helpful for normal duties like textual content classification.
# Notice: Requires transformers and datasets libraries
# pip set up transformers datasets torch # or tensorflow/jax
import consider
from consider import evaluator
from transformers import pipeline
from datasets import load_dataset
# Load a pre-trained textual content classification pipeline
# Utilizing a smaller mannequin for doubtlessly sooner execution
strive:
pipe = pipeline("text-classification", mannequin="distilbert-base-uncased-finetuned-sst-2-english", system=-1) # Use CPU
besides Exception as e:
print(f"Couldn't load pipeline: {e}")
pipe = None
if pipe:
# Load a small subset of the IMDB dataset
strive:
information = load_dataset("imdb", break up="check").shuffle(seed=42).choose(vary(100)) # Smaller subset for pace
besides Exception as e:
print(f"Couldn't load dataset: {e}")
information = None
if information:
# Load the accuracy metric
accuracy_metric = consider.load("accuracy")
# Create an evaluator for the duty
task_evaluator = evaluator("text-classification")
# Right label_mapping for IMDB dataset
label_mapping = {
'NEGATIVE': 0, # Map NEGATIVE to 0
'POSITIVE': 1 # Map POSITIVE to 1
}
# Compute outcomes
eval_results = task_evaluator.compute(
model_or_pipeline=pipe,
information=information,
metric=accuracy_metric,
input_column="textual content", # Specify the textual content column
label_column="label", # Specify the label column
label_mapping=label_mapping # Cross the corrected label mapping
)
print("nEvaluator Outcomes:")
print(eval_results)
# Compute with bootstrapping for confidence intervals
bootstrap_results = task_evaluator.compute(
model_or_pipeline=pipe,
information=information,
metric=accuracy_metric,
input_column="textual content",
label_column="label",
label_mapping=label_mapping, # Cross the corrected label mapping
technique="bootstrap",
n_resamples=10 # Use fewer resamples for sooner demo
)
print("nEvaluator Outcomes with Bootstrapping:")
print(bootstrap_results)
Output:
Gadget set to make use of cpuEvaluator Outcomes:
{'accuracy': 0.9, 'total_time_in_seconds': 24.277618517999997,
'samples_per_second': 4.119020155368932, 'latency_in_seconds':
0.24277618517999996}Evaluator Outcomes with Bootstrapping:
{'accuracy': {'confidence_interval': (np.float64(0.8703044820750653),
np.float64(0.9335706530476571)), 'standard_error':
np.float64(0.02412928142780514), 'rating': 0.9}, 'total_time_in_seconds':
23.871316319000016, 'samples_per_second': 4.189128017226537,
'latency_in_seconds': 0.23871316319000013}
Clarification:
- We load a transformers pipeline for textual content classification and a pattern of the IMDb dataset.
- We create an evaluator particularly for “text-classification”.
- The compute technique handles feeding information (textual content column) to the pipeline, getting predictions, evaluating them to the true labels (label column) utilizing the required metric, and making use of the label_mapping.
- It returns the metric rating together with efficiency stats like complete time and samples per second.
- Utilizing technique=”bootstrap” performs resampling to estimate confidence intervals and commonplace error for the metric, giving a way of the rating’s stability.
Utilizing Analysis Suites
Analysis Suites bundle a number of evaluations, usually concentrating on particular benchmarks like GLUE. This enables working a mannequin towards an ordinary set of duties.
# Notice: Operating a full suite may be computationally intensive and time-consuming.
# This instance demonstrates the idea however would possibly take a very long time or require vital assets.
# It additionally installs a number of datasets and will require particular mannequin configurations.
import consider
strive:
print("nLoading GLUE analysis suite (this would possibly obtain datasets)...")
# Load the GLUE process straight
# Utilizing "mrpc" for instance process, however you possibly can select from the legitimate ones listed above
process = consider.load("glue", "mrpc") # Specify the duty like "mrpc", "sst2", and many others.
print("Process loaded.")
# Now you can run the duty on a mannequin (for instance: "distilbert-base-uncased")
# WARNING: This would possibly take time for inference or fine-tuning.
# outcomes = process.compute(model_or_pipeline="distilbert-base-uncased")
# print("nEvaluation Outcomes (MRPC Process):")
# print(outcomes)
print("Skipping mannequin inference for brevity on this instance.")
print("Confer with Hugging Face documentation for full EvaluationSuite utilization.")
besides Exception as e:
print(f"Couldn't load or run analysis suite: {e}")
Output:
Loading GLUE analysis suite (this would possibly obtain datasets)...Process loaded.
Skipping mannequin inference for brevity on this instance.
Confer with Hugging Face documentation for full EvaluationSuite utilization.
Clarification:
- EvaluationSuite.load masses a predefined set of analysis duties (right here, simply the MRPC process from the GLUE benchmark for demonstration).
- The suite.run(“model_name”) command would sometimes execute the mannequin on every dataset throughout the suite and compute the related metrics.
- The output is normally a listing of dictionaries, every containing the outcomes for one process within the suite. (Notice: Operating this usually requires particular atmosphere setups and substantial compute time).
Visualizing Analysis Outcomes
Visualizations assist evaluate a number of fashions throughout completely different metrics. Radar plots are efficient for this.
import consider
import matplotlib.pyplot as plt # Guarantee matplotlib is put in
from consider.visualization import radar_plot
# Pattern information for a number of fashions throughout a number of metrics
# Decrease latency is healthier, so we'd invert it or think about it individually.
information = [
{"accuracy": 0.99, "precision": 0.80, "f1": 0.95, "latency_inv": 1/33.6},
{"accuracy": 0.98, "precision": 0.87, "f1": 0.91, "latency_inv": 1/11.2},
{"accuracy": 0.98, "precision": 0.78, "f1": 0.88, "latency_inv": 1/87.6},
{"accuracy": 0.88, "precision": 0.78, "f1": 0.81, "latency_inv": 1/101.6}
]
model_names = ["Model A", "Model B", "Model C", "Model D"]
# Generate the radar plot
# Greater values are usually higher on a radar plot
strive:
# Generate radar plot (make sure you cross an accurate format and that information is legitimate)
plot = radar_plot(information=information, model_names=model_names)
# Show the plot
plt.present() # Explicitly present the plot, is perhaps essential in some environments
# To save lots of the plot to a file (uncomment to make use of)
# plot.savefig("model_comparison_radar.png")
plt.shut() # Shut the plot window after exhibiting/saving
besides ImportError:
print("Visualization requires matplotlib. Run: pip set up matplotlib")
besides Exception as e:
print(f"Couldn't generate plot: {e}")
Output:
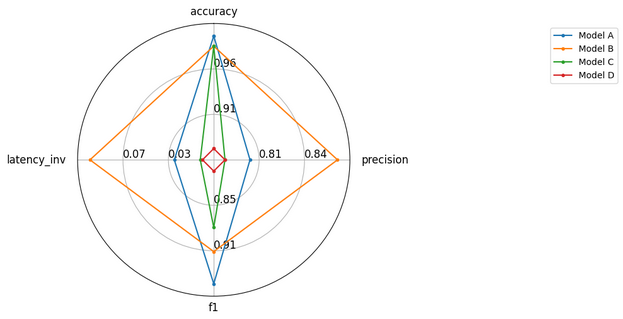
Clarification:
- We put together pattern outcomes for 4 fashions throughout accuracy, precision, F1, and inverted latency (so increased is healthier).
- radar_plot creates a plot the place every axis represents a metric, exhibiting how fashions evaluate visually.
Saving Analysis Outcomes
It can save you your analysis outcomes to a file, usually in JSON format, for record-keeping or later evaluation.
import consider
from pathlib import Path
# Carry out an analysis
accuracy_metric = consider.load("accuracy")
end result = accuracy_metric.compute(references=[0, 1, 0, 1], predictions=[1, 0, 0, 1])
print(f"Outcome to save lots of: {end result}")
# Outline hyperparameters or different metadata
hyperparams = {"model_name": "my_custom_model", "learning_rate": 0.001}
run_details = {"experiment_id": "run_42"}
# Mix outcomes and metadata
save_data = {**end result, **hyperparams, **run_details}
# Outline save listing and filename
save_dir = Path("./evaluation_results")
save_dir.mkdir(exist_ok=True) # Create listing if it does not exist
# Use consider.save to retailer the outcomes
strive:
saved_path = consider.save(save_directory=save_dir, **save_data)
print(f"Outcomes saved to: {saved_path}")
# You too can manually save as JSON
import json
manual_save_path = save_dir / "manual_results.json"
with open(manual_save_path, 'w') as f:
json.dump(save_data, f, indent=4)
print(f"Outcomes manually saved to: {manual_save_path}")
besides Exception as e:
# Catch potential git-related errors if run outdoors a repo
print(f"consider.save encountered a difficulty (probably git associated): {e}")
print("Making an attempt guide JSON save as a substitute.")
import json
manual_save_path = save_dir / "manual_results_fallback.json"
with open(manual_save_path, 'w') as f:
json.dump(save_data, f, indent=4)
print(f"Outcomes manually saved to: {manual_save_path}")
Output:
Outcome to save lots of: {'accuracy': 0.5}consider.save encountered a difficulty (probably git associated): save() lacking 1
required positional argument: 'path_or_file'Making an attempt guide JSON save as a substitute.
Outcomes manually saved to: evaluation_results/manual_results_fallback.json
Clarification:
- We mix the computed end result dictionary with different metadata like hyperparams.
- consider.save makes an attempt to save lots of this information to a JSON file within the specified listing. It’d attempt to add git commit data if run inside a repository, which might trigger errors in any other case (as seen within the unique log).
- We embrace a fallback to manually save the dictionary as a JSON file, which is commonly enough.
Selecting the Proper Metric
Choosing the suitable metric is essential. Contemplate these factors:
- Process Kind: Is it classification, translation, summarization, NER, QA? Use metrics commonplace for that process (Accuracy/F1 for classification, BLEU/ROUGE for technology, Seqeval for NER, SQuAD for QA).
- Dataset: Some benchmarks (like GLUE, SQuAD) have particular related metrics. Leaderboards (e.g., on Papers With Code) usually present generally used metrics for particular datasets.
- Purpose: What facet of efficiency issues most?
- Accuracy: Total correctness (good for balanced lessons).
- Precision/Recall/F1: Essential for imbalanced lessons or when false positives/negatives have completely different prices.
- BLEU/ROUGE: Fluency and content material overlap in textual content technology.
- Perplexity: How properly a language mannequin predicts a pattern (decrease is healthier, usually used for generative fashions).
- Metric Playing cards: Learn the Hugging Face metric playing cards (documentation) for detailed explanations, limitations, and applicable use circumstances (e.g., BLEU card, SQuAD card).
Conclusion
The Hugging Face Consider library gives a flexible and user-friendly method to assess massive language fashions and datasets. It gives commonplace metrics, dataset measurements, and instruments just like the Evaluator and EvaluationSuite to streamline the method. By utilizing these instruments and selecting metrics applicable in your process, you possibly can achieve clear insights into your mannequin’s strengths and weaknesses.
For extra particulars and superior utilization, seek the advice of the official assets:
Login to proceed studying and revel in expert-curated content material.