As a library developer, you might create a well-liked utility that lots of of
1000’s of builders depend on each day, akin to lodash or React. Over time,
utilization patterns would possibly emerge that transcend your preliminary design. When this
occurs, you might want to increase an API by including parameters or modifying
perform signatures to repair edge circumstances. The problem lies in rolling out
these breaking modifications with out disrupting your customers’ workflows.
That is the place codemods are available—a strong device for automating
large-scale code transformations, permitting builders to introduce breaking
API modifications, refactor legacy codebases, and keep code hygiene with
minimal handbook effort.
On this article, we’ll discover what codemods are and the instruments you possibly can
use to create them, akin to jscodeshift, hypermod.io, and codemod.com. We’ll stroll by way of real-world examples,
from cleansing up characteristic toggles to refactoring element hierarchies.
You’ll additionally discover ways to break down advanced transformations into smaller,
testable items—a observe often known as codemod composition—to make sure
flexibility and maintainability.
By the tip, you’ll see how codemods can grow to be an important a part of your
toolkit for managing large-scale codebases, serving to you retain your code clear
and maintainable whereas dealing with even probably the most difficult refactoring
duties.
Breaking Adjustments in APIs
Returning to the situation of the library developer, after the preliminary
launch, new utilization patterns emerge, prompting the necessity to lengthen an
For easy modifications, a fundamental find-and-replace within the IDE would possibly work. In
extra advanced circumstances, you would possibly resort to utilizing instruments like sed
or awk
. Nonetheless, when your library is broadly adopted, the
scope of such modifications turns into tougher to handle. You may’t ensure how
extensively the modification will impression your customers, and the very last thing
you need is to interrupt current performance that doesn’t want
updating.
A standard strategy is to announce the breaking change, launch a brand new
model, and ask customers emigrate at their very own tempo. However this workflow,
whereas acquainted, usually does not scale effectively, particularly for main shifts.
Think about React’s transition from class parts to perform parts
with hooks—a paradigm shift that took years for giant codebases to completely
undertake. By the point groups managed emigrate, extra breaking modifications have been
usually already on the horizon.
For library builders, this case creates a burden. Sustaining
a number of older variations to help customers who haven’t migrated is each
expensive and time-consuming. For customers, frequent modifications danger eroding belief.
They might hesitate to improve or begin exploring extra steady options,
which perpetuating the cycle.
However what in case you may assist customers handle these modifications robotically?
What in case you may launch a device alongside your replace that refactors
their code for them—renaming capabilities, updating parameter order, and
eradicating unused code with out requiring handbook intervention?
That’s the place codemods are available. A number of libraries, together with React
and Subsequent.js, have already embraced codemods to clean the trail for model
bumps. For instance, React supplies codemods to deal with the migration from
older API patterns, just like the outdated Context API, to newer ones.
So, what precisely is the codemod we’re speaking about right here?
What’s a Codemod?
A codemod (code modification) is an automatic script used to rework
code to observe new APIs, syntax, or coding requirements. Codemods use
Summary Syntax Tree (AST) manipulation to use constant, large-scale
modifications throughout codebases. Initially developed at Fb, codemods helped
engineers handle refactoring duties for giant tasks like React. As
Fb scaled, sustaining the codebase and updating APIs turned
more and more troublesome, prompting the event of codemods.
Manually updating 1000’s of information throughout completely different repositories was
inefficient and error-prone, so the idea of codemods—automated scripts
that remodel code—was launched to sort out this drawback.
The method sometimes entails three primary steps:
- Parsing the code into an AST, the place every a part of the code is
represented as a tree construction. - Modifying the tree by making use of a change, akin to renaming a
perform or altering parameters. - Rewriting the modified tree again into the supply code.
By utilizing this strategy, codemods be sure that modifications are utilized
constantly throughout each file in a codebase, decreasing the possibility of human
error. Codemods also can deal with advanced refactoring situations, akin to
modifications to deeply nested constructions or eradicating deprecated API utilization.
If we visualize the method, it will look one thing like this:
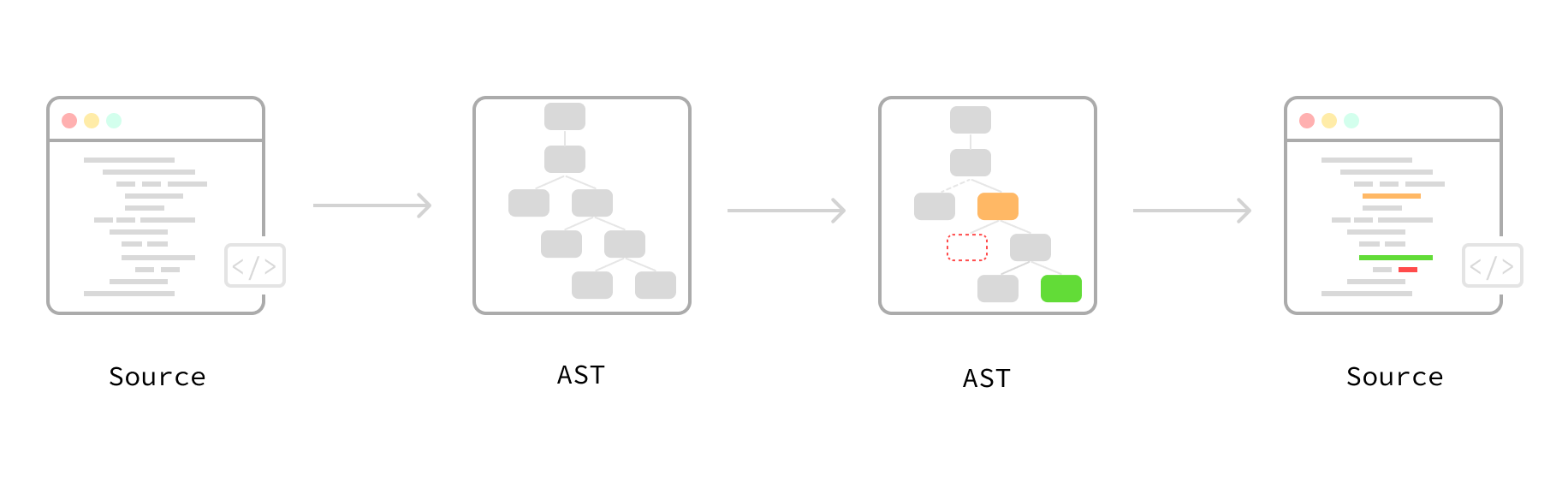
Determine 1: The three steps of a typical codemod course of
The concept of a program that may “perceive” your code after which carry out
automated transformations isn’t new. That’s how your IDE works once you
run refactorings like
Basically, your IDE parses the supply code into ASTs and applies
predefined transformations to the tree, saving the consequence again into your
information.
For contemporary IDEs, many issues occur underneath the hood to make sure modifications
are utilized accurately and effectively, akin to figuring out the scope of
the change and resolving conflicts like variable identify collisions. Some
refactorings even immediate you to enter parameters, akin to when utilizing
order of parameters or default values earlier than finalizing the change.
Use jscodeshift in JavaScript Codebases
Let’s have a look at a concrete instance to grasp how we may run a
codemod in a JavaScript undertaking. The JavaScript group has a number of
instruments that make this work possible, together with parsers that convert supply
code into an AST, in addition to transpilers that may remodel the tree into
different codecs (that is how TypeScript works). Moreover, there are
instruments that assist apply codemods to whole repositories robotically.
One of the in style instruments for writing codemods is jscodeshift, a toolkit maintained by Fb.
It simplifies the creation of codemods by offering a strong API to
manipulate ASTs. With jscodeshift, builders can seek for particular
patterns within the code and apply transformations at scale.
You should utilize jscodeshift
to establish and change deprecated API calls
with up to date variations throughout a whole undertaking.
Let’s break down a typical workflow for composing a codemod
manually.
Clear a Stale Function Toggle
Let’s begin with a easy but sensible instance to show the
energy of codemods. Think about you’re utilizing a characteristic
toggle in your
codebase to manage the discharge of unfinished or experimental options.
As soon as the characteristic is reside in manufacturing and dealing as anticipated, the following
logical step is to wash up the toggle and any associated logic.
As an illustration, contemplate the next code:
const knowledge = featureToggle('feature-new-product-list') ? { identify: 'Product' } : undefined;
As soon as the characteristic is totally launched and not wants a toggle, this
could be simplified to:
const knowledge = { identify: 'Product' };
The duty entails discovering all cases of featureToggle
within the
codebase, checking whether or not the toggle refers to
feature-new-product-list
, and eradicating the conditional logic surrounding
it. On the similar time, different characteristic toggles (like
feature-search-result-refinement
, which can nonetheless be in improvement)
ought to stay untouched. The codemod must perceive the construction
of the code to use modifications selectively.
Understanding the AST
Earlier than we dive into writing the codemod, let’s break down how this
particular code snippet seems to be in an AST. You should utilize instruments like AST
Explorer to visualise how supply code and AST
are mapped. It’s useful to grasp the node varieties you are interacting
with earlier than making use of any modifications.
The picture beneath exhibits the syntax tree when it comes to ECMAScript syntax. It
incorporates nodes like Identifier
(for variables), StringLiteral
(for the
toggle identify), and extra summary nodes like CallExpression
and
ConditionalExpression
.
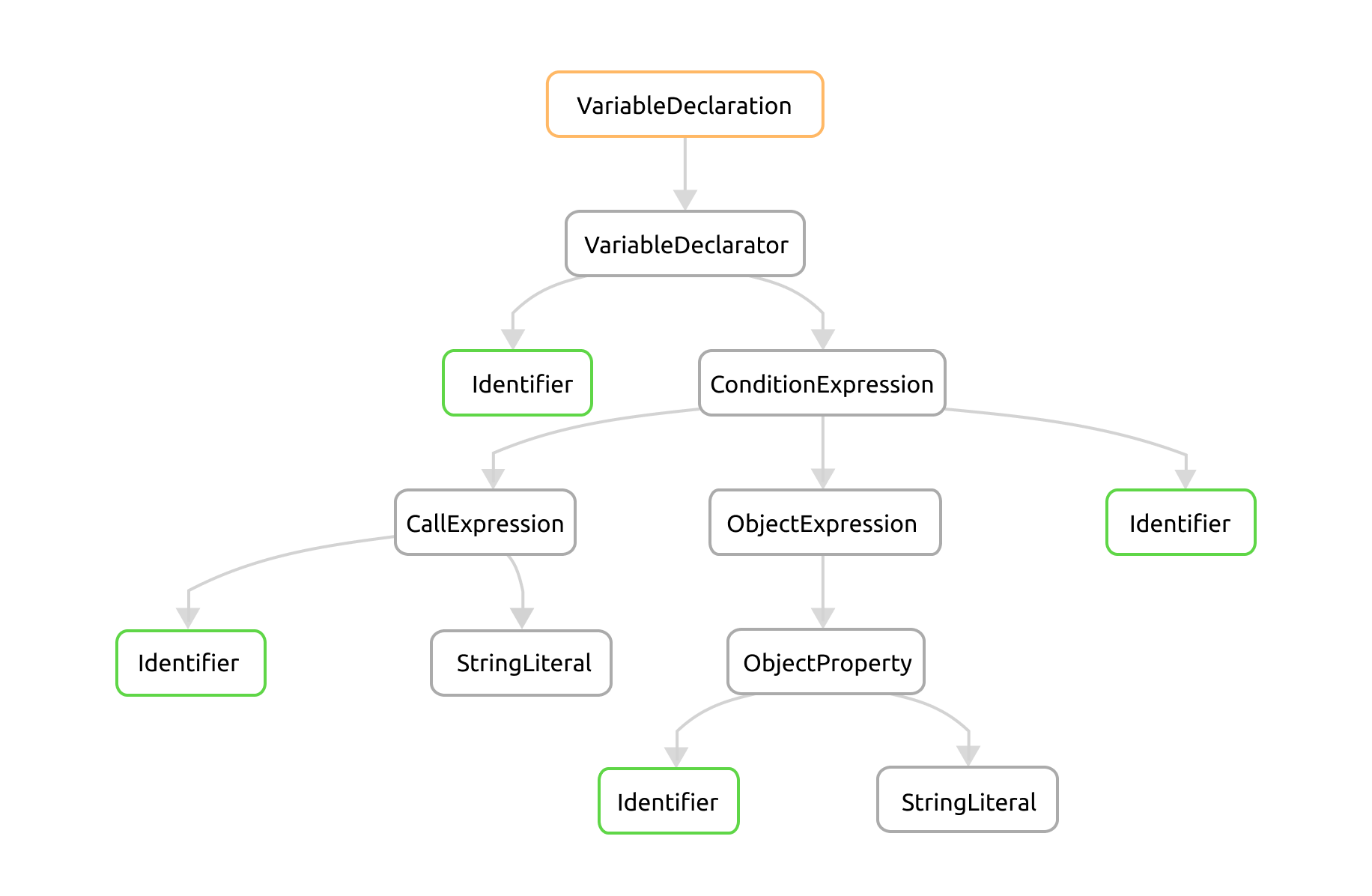
Determine 2: The Summary Syntax Tree illustration of the characteristic toggle verify
On this AST illustration, the variable knowledge
is assigned utilizing a
ConditionalExpression
. The take a look at a part of the expression calls
featureToggle('feature-new-product-list')
. If the take a look at returns true
,
the consequent department assigns { identify: 'Product' }
to knowledge
. If
false
, the alternate department assigns undefined
.
For a activity with clear enter and output, I choose writing exams first,
then implementing the codemod. I begin by defining a unfavorable case to
guarantee we don’t unintentionally change issues we wish to depart untouched,
adopted by an actual case that performs the precise conversion. I start with
a easy situation, implement it, then add a variation (like checking if
featureToggle is named inside an if assertion), implement that case, and
guarantee all exams move.
This strategy aligns effectively with Take a look at-Pushed Improvement (TDD), even
in case you don’t observe TDD commonly. Realizing precisely what the
transformation’s inputs and outputs are earlier than coding improves security and
effectivity, particularly when tweaking codemods.
With jscodeshift, you possibly can write exams to confirm how the codemod
behaves:
const remodel = require("../remove-feature-new-product-list"); defineInlineTest( remodel, {}, ` const knowledge = featureToggle('feature-new-product-list') ? { identify: 'Product' } : undefined; `, ` const knowledge = { identify: 'Product' }; `, "delete the toggle feature-new-product-list in conditional operator" );
The defineInlineTest
perform from jscodeshift lets you outline
the enter, anticipated output, and a string describing the take a look at’s intent.
Now, working the take a look at with a traditional jest
command will fail as a result of the
codemod isn’t written but.
The corresponding unfavorable case would make sure the code stays unchanged
for different characteristic toggles:
defineInlineTest( remodel, {}, ` const knowledge = featureToggle('feature-search-result-refinement') ? { identify: 'Product' } : undefined; `, ` const knowledge = featureToggle('feature-search-result-refinement') ? { identify: 'Product' } : undefined; `, "don't change different characteristic toggles" );
Writing the Codemod
Let’s begin by defining a easy remodel perform. Create a file
referred to as remodel.js
with the next code construction:
module.exports = perform(fileInfo, api, choices) { const j = api.jscodeshift; const root = j(fileInfo.supply); // manipulate the tree nodes right here return root.toSource(); };
This perform reads the file right into a tree and makes use of jscodeshift’s API to
question, modify, and replace the nodes. Lastly, it converts the AST again to
supply code with .toSource()
.
Now we are able to begin implementing the remodel steps:
- Discover all cases of
featureToggle
. - Confirm that the argument handed is
'feature-new-product-list'
. - Change the complete conditional expression with the consequent half,
successfully eradicating the toggle.
Right here’s how we obtain this utilizing jscodeshift
:
module.exports = perform (fileInfo, api, choices) { const j = api.jscodeshift; const root = j(fileInfo.supply); // Discover ConditionalExpression the place the take a look at is featureToggle('feature-new-product-list') root .discover(j.ConditionalExpression, { take a look at: { callee: { identify: "featureToggle" }, arguments: [{ value: "feature-new-product-list" }], }, }) .forEach((path) => { // Change the ConditionalExpression with the 'consequent' j(path).replaceWith(path.node.consequent); }); return root.toSource(); };
The codemod above:
- Finds
ConditionalExpression
nodes the place the take a look at calls
featureToggle('feature-new-product-list')
. - Replaces the complete conditional expression with the resultant (i.e.,
{
), eradicating the toggle logic and leaving simplified code
identify: 'Product' }
behind.
This instance demonstrates how straightforward it’s to create a helpful
transformation and apply it to a big codebase, considerably decreasing
handbook effort.
You’ll want to write down extra take a look at circumstances to deal with variations like
if-else
statements, logical expressions (e.g.,
!featureToggle('feature-new-product-list')
), and so forth to make the
codemod sturdy in real-world situations.
As soon as the codemod is prepared, you possibly can try it out on a goal codebase,
such because the one you are engaged on. jscodeshift supplies a command-line
device that you should use to use the codemod and report the outcomes.
$ jscodeshift -t transform-name src/
After validating the outcomes, verify that every one practical exams nonetheless
move and that nothing breaks—even in case you’re introducing a breaking change.
As soon as happy, you possibly can commit the modifications and lift a pull request as
a part of your regular workflow.
Codemods Enhance Code High quality and Maintainability
Codemods aren’t simply helpful for managing breaking API modifications—they’ll
considerably enhance code high quality and maintainability. As codebases
evolve, they usually accumulate technical debt, together with outdated characteristic
toggles, deprecated strategies, or tightly coupled parts. Manually
refactoring these areas could be time-consuming and error-prone.
By automating refactoring duties, codemods assist preserve your codebase clear
and freed from legacy patterns. Frequently making use of codemods lets you
implement new coding requirements, take away unused code, and modernize your
codebase with out having to manually modify each file.