The world of AI and Giant Language Fashions (LLMs) strikes shortly. Integrating exterior instruments and real-time knowledge is important for constructing actually highly effective purposes. The Mannequin Context Protocol (MCP) gives a normal method to bridge this hole. This information offers a transparent, beginner-friendly walkthrough for creating an MCP shopper server utilizing LangChain. Understanding the MCP shopper server structure helps construct strong AI brokers. We’ll cowl the necessities, together with what’s MCP server performance, and supply a sensible MCP shopper server utilizing LangChain instance.
Understanding the Mannequin Context Protocol (MCP)
So, what’s MCP server and shopper interplay all about? The Mannequin Context Protocol (MCP) is an open-standard system. Anthropic developed it to attach LLMs with exterior instruments and knowledge sources successfully. It makes use of a structured and reusable strategy. MCP helps AI fashions discuss to totally different techniques. This permits them to entry present data and do duties past their preliminary coaching. Consider it as a common translator between the AI and the surface world, forming the core of the MCP shopper server structure.
Key Options of MCP
MCP stands out attributable to a number of vital options:
- Standardized Integration: MCP provides a single, constant method to join LLMs to many instruments and knowledge sources. This removes the necessity for distinctive code for each connection. It simplifies the MCP shopper server utilizing LangChain setup.
- Context Administration: The protocol ensures the AI mannequin retains observe of the dialog context throughout a number of steps. This prevents shedding vital data when duties require a number of interactions.
- Safety and Isolation: MCP contains robust safety measures. It controls entry strictly and retains server connections separate utilizing permission boundaries. This ensures secure communication between the shopper and server.
Position of MCP in LLM-Based mostly Functions
LLM purposes usually want outdoors knowledge. They may want to question databases, fetch paperwork, or use internet APIs. MCP acts as an important center layer. It lets fashions work together with these exterior sources easily, without having guide steps. Utilizing an MCP shopper server utilizing LangChain lets builders construct smarter AI brokers. These brokers turn into extra succesful, work sooner, and function securely inside a well-defined MCP shopper server structure. This setup is prime for superior AI assistants. Now Let’s take a look at the implementation half.
Setting Up the Surroundings
Earlier than constructing our MCP shopper server utilizing LangChain, let’s put together the setting. You want these things:
- Python model 3.11 or newer.
- Arrange a brand new digital setting (optionally available)
- An API key (e.g., OpenAI or Groq, relying on the mannequin you select).
- Particular Python libraries: langchain-mcp-adapters, langgraph, and an LLM library (like langchain-openai or langchain-groq) of your selection.
Set up the wanted libraries utilizing pip. Open your terminal or command immediate and run:
pip set up langchain-mcp-adapters langgraph langchain-groq # Or langchain-openai
Be sure to have the proper Python model and essential keys prepared.
Constructing the MCP Server
The MCP server’s job is to supply instruments the shopper can use. In our MCP shopper server utilizing langchain instance, we’ll construct a easy server. This server will deal with fundamental math operations in addition to advanced climate api to get climate particulars of a metropolis. Understanding what’s MCP server performance begins right here.
Create a Python file named mcp_server.py:
- Let’s import the required libraries
import math
import requests
from mcp.server.fastmcp import FastMCP
2. Initialize the FastMCP object
mcp= FastMCP("Math")
3. Let’s outline the mathematics instruments
@mcp.software()
def add(a: int, b: int) -> int:
print(f"Server obtained add request: {a}, {b}")
return a + b
@mcp.software()
def multiply(a: int, b: int) -> int:
print(f"Server obtained multiply request: {a}, {b}")
return a * b
@mcp.software()
def sine(a: int) -> int:
print(f"Server obtained sine request: {a}")
return math.sin(a)
4. Now, Let’s outline a climate software, ensure you have API from right here.
WEATHER_API_KEY = "YOUR_API_KEY"
@mcp.software()
def get_weather(metropolis: str) -> dict:
"""
Fetch present climate for a given metropolis utilizing WeatherAPI.com.
Returns a dictionary with metropolis, temperature (C), and situation.
"""
print(f"Server obtained climate request: {metropolis}")
url = f"http://api.weatherapi.com/v1/present.json?key={WEATHER_API_KEY}&q={metropolis}"
response = requests.get(url)
if response.status_code != 200:
return {"error": f"Did not fetch climate for {metropolis}."}
knowledge = response.json()
return {
"metropolis": knowledge["location"]["name"],
"area": knowledge["location"]["region"],
"nation": knowledge["location"]["country"],
"temperature_C": knowledge["current"]["temp_c"],
"situation": knowledge["current"]["condition"]["text"]
}
5. Now, instantiate the mcp server
if __name__ =="__main__":
print("Beginning MCP Server....")
mcp.run(transport="stdio")
Clarification:
This script units up a easy MCP server named “Math”. It makes use of FastMCP to outline 4 instruments, add, multiply, sine and get_weather marked by the @mcp.software() decorator. Kind hints inform MCP in regards to the anticipated inputs and outputs. The server runs utilizing commonplace enter/output (stdio) for communication when executed instantly. This demonstrates what’s MCP server in a fundamental setup.
Run the server: Open your terminal and navigate to the listing containing mcp_server.py. Then run:
python mcp_server.py
The server ought to begin with none warnings. This server will carry on operating for the shopper to entry the instruments
Output:

Constructing the MCP Shopper
The shopper connects to the server, sends requests (like asking the agent to carry out a calculation and fetch the reside climate), and handles the responses. This demonstrates the shopper facet of the MCP shopper server utilizing LangChain.
Create a Python file named shopper.py:
- Import the mandatory libraries first
# shopper.py
from mcp import ClientSession, StdioServerParameters
from mcp.shopper.stdio import stdio_client
from langchain_mcp_adapters.instruments import load_mcp_tools
from langgraph.prebuilt import create_react_agent
from langchain_groq import ChatGroq
from langchain_openai import ChatOpenAI
import asyncio
import os
- Arrange the API key for the LLM (Groq or OpenAI) and initialize the LLM mannequin
# Set your API key (exchange along with your precise key or use setting variables)
GROQ_API_KEY = "YOUR_GROQ_API_KEY" # Change along with your key
os.environ["GROQ_API_KEY"] = GROQ_API_KEY
# OPENAI_API_KEY = "YOUR_OPENAI_API_KEY"
# os.environ["OPENAI_API_KEY"] = OPENAI_API_KEY
# Initialize the LLM mannequin
mannequin = ChatGroq(mannequin="llama3-8b-8192", temperature=0)
# mannequin = ChatOpenAI(mannequin="gpt-4o-mini", temperature=0)
- Now, outline the parameters to begin the MCP server course of.
server_params = StdioServerParameters(
command="python", # Command to execute
args=["mcp_server.py"] # Arguments for the command (our server script)
)
- Let’s outline the Asynchronous operate to run the agent interplay
async def run_agent():
async with stdio_client(server_params) as (learn, write):
async with ClientSession(learn, write) as session:
await session.initialize()
print("MCP Session Initialized.")
instruments = await load_mcp_tools(session)
print(f"Loaded Instruments: {[tool.name for tool in tools]}")
agent = create_react_agent(mannequin, instruments)
print("ReAct Agent Created.")
print(f"Invoking agent with question")
response = await agent.ainvoke({
"messages": [("user", "What is (7+9)x17, then give me sine of the output recieved and then tell me What's the weather in Torronto, Canada?")]
})
print("Agent invocation full.")
# Return the content material of the final message (often the agent's closing reply)
return response["messages"][-1].content material
- Now, run this operate and look ahead to the outcomes on th terminal
# Commonplace Python entry level verify
if __name__ == "__main__":
# Run the asynchronous run_agent operate and look ahead to the consequence
print("Beginning MCP Shopper...")
consequence = asyncio.run(run_agent())
print("nAgent Closing Response:")
print(consequence)
Clarification:
This shopper script configures an LLM (utilizing ChatGroq right here; bear in mind to set your API key). It defines learn how to begin the server utilizing StdioServerParameters. The run_agent operate connects to the server by way of stdio_client, creates a ClientSession, and initializes it. load_mcp_tools fetches the server’s instruments for LangChain. A create_react_agent makes use of the LLM and instruments to course of a person question. Lastly, agent.ainvoke sends the question, letting the agent probably use the server’s instruments to search out the reply. This reveals a whole MCP shopper server utilizing langchain instance.
Run the shopper:
python shopper.py
Output:
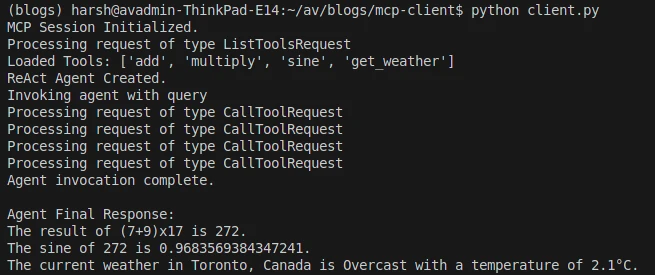
We will see that the shopper begins the server course of, initializes the connection, hundreds instruments, invokes the agent, and prints the ultimate reply calculated by calling the server’s add software additionally known as climate api and retrieving the reside climate knowledge.
Actual-World Functions
Utilizing an MCP shopper server utilizing LangChain opens up many potentialities for creating subtle AI brokers. Some sensible purposes embody:
- LLM Independency: By using Langchain, we are able to now combine any LLM with MCP. Beforehand we have been
- Knowledge Retrieval: Brokers can connect with database servers by way of MCP to fetch real-time buyer knowledge or question inside data bases.
- Doc Processing: An agent may use MCP instruments to work together with a doc administration system, permitting it to summarize, extract data, or replace paperwork based mostly on person requests.
- Job Automation: Combine with varied enterprise techniques (like CRMs, calendars, or undertaking administration instruments) by way of MCP servers to automate routine duties like scheduling conferences or updating gross sales data. The MCP shopper server structure helps these advanced workflows.
Greatest Practices
When constructing your MCP shopper server utilizing LangChain, observe good practices for higher outcomes:
- Undertake a modular design by creating particular instruments for distinct duties and holding server logic separate from shopper logic.
- Implement strong error dealing with in each server instruments and the shopper agent so the system can handle failures gracefully.
- Prioritize safety, particularly if the server handles delicate knowledge, by utilizing MCP’s options like entry controls and permission boundaries.
- Present clear descriptions and docstrings on your MCP instruments; this helps the agent perceive their goal and utilization.
Widespread Pitfalls
Be conscious of potential points when growing your system. Context loss can happen in advanced conversations if the agent framework doesn’t handle state correctly, resulting in errors. Poor useful resource administration in long-running MCP servers may trigger reminiscence leaks or efficiency degradation, so deal with connections and file handles fastidiously. Guarantee compatibility between the shopper and server transport mechanisms, as mismatches (like one utilizing stdio and the opposite anticipating HTTP) will stop communication. Lastly, look ahead to software schema mismatches the place the server software’s definition doesn’t align with the shopper’s expectation, which might block software execution. Addressing these factors strengthens your MCP shopper server utilizing LangChain implementation.
Conclusion
Leveraging the Mannequin Context Protocol with LangChain offers a robust and standardized method to construct superior AI brokers. By creating an MCP shopper server utilizing LangChain, you allow your LLMs to work together securely and successfully with exterior instruments and knowledge sources. This information demonstrated a fundamental MCP shopper server utilizing LangChain instance, outlining the core MCP shopper server structure and what’s MCP server performance entails. This strategy simplifies integration, boosts agent capabilities, and ensures dependable operations, paving the way in which for extra clever and helpful AI purposes.
Steadily Requested Questions
A. MCP is an open commonplace designed by Anthropic. It offers a structured manner for Giant Language Fashions (LLMs) to work together with exterior instruments and knowledge sources securely.
A. LangChain offers the framework for constructing brokers, whereas MCP gives a standardized protocol for software communication. Combining them simplifies constructing brokers that may reliably use exterior capabilities.
A. MCP is designed to be transport-agnostic. Widespread implementations use commonplace enter/output (stdio) for native processes or HTTP-based Server-Despatched Occasions (SSE) for community communication.
A. Sure, MCP is designed with safety in thoughts. It contains options like permission boundaries and connection isolation to make sure safe interactions between shoppers and servers.
A. Completely. LangChain helps many LLM suppliers. So long as the chosen LLM works with LangChain/LangGraph agent frameworks, it could work together with instruments loaded by way of an MCP shopper.
Login to proceed studying and luxuriate in expert-curated content material.