Within the fast-paced world of educational analysis, effectively gathering, synthesizing, and presenting data is essential. Manually sourcing and summarizing literature could be tedious, diverting researchers from deeper evaluation and discovery. That is the place a Multi-Agent Analysis Assistant System utilizing Pydantic is available in—an clever structure the place specialised brokers collaborate to deal with advanced duties with modularity and scalability. Nonetheless, managing a number of brokers introduces challenges in information consistency, validation, and structured communication. Multi-Agent Analysis Assistant Techniques utilizing Pydantic present an answer by implementing clear information schemas, making certain strong dealing with, and decreasing system complexity.
On this weblog, we’ll stroll by constructing a structured multi-agent analysis assistant utilizing Pydantic, integrating instruments like Pydantic-ai and arxiv, with step-by-step code explanations and anticipated outcomes.
Studying Aims
- Perceive the position of structured information modeling in a Multi-Agent Analysis Assistant System utilizing Pydantic to make sure dependable and constant communication amongst clever brokers.
- Outline and implement clear, structured information schemas utilizing Multi-Agent Analysis Assistant Techniques utilizing Pydantic for seamless integration, modular agent orchestration, and environment friendly automated analysis workflows.
- Design and orchestrate modular brokers, every liable for particular duties comparable to question refinement, information retrieval, key phrase extraction, and summarization.
- Combine exterior APIs (like arXiv) seamlessly into automated workflows utilizing structured agent interactions.
- Generate professional-quality outputs (e.g., PDF reviews) immediately from structured agent outputs, considerably enhancing the sensible usability of your automated analysis workflows.
This text was printed as part of the Information Science Blogathon.
Defining Clear Information Fashions with Pydantic
In multi-agent methods, clearly outlined structured information fashions are foundational. When a number of clever brokers work together, every agent is dependent upon receiving and sending well-defined, predictable information. And not using a structured schema, even minor inconsistencies can result in system-wide errors which might be notoriously tough to debug.
Utilizing Pydantic, we are able to deal with this problem elegantly. Pydantic gives a easy but highly effective solution to outline information schemas in Python. It ensures information consistency, considerably reduces potential runtime bugs, and facilitates seamless validation at each step of an agent’s workflow.
Beneath is a sensible instance of defining structured information fashions utilizing Pydantic, which our brokers will use for clear communication:
from pydantic import BaseModel, Subject
class PaperMetadata(BaseModel):
title: str = Subject(..., description="Title of the paper")
summary: str = Subject(..., description="Summary of the paper")
authors: listing[str] = Subject(..., description="Checklist of authors")
publication_date: str = Subject(..., description="Publication date")
Clarification of Every Subject
- title : The title of the retrieved analysis paper. It’s important for fast reference, organizing, and show functions by numerous brokers.
- summary : Accommodates a concise abstract or summary supplied by the paper’s authors. This summary is essential for key phrase extraction and summarization brokers.
- authors : Lists the authors of the paper. This metadata can help in additional queries, author-specific analyses, or quotation monitoring.
- publication_date : Represents the date the paper was printed or submitted. That is vital for sorting, filtering, and making certain recency in analysis.
Every agent in our multi-agent system depends on these structured fields. Let’s briefly introduce the brokers we’ll construct round this information mannequin. In our system, we’ll design 5 specialised brokers:
- Immediate Processor Agent
- Paper Retrieval Agent
- Key phrase Extraction Agent
- Summarization Agent
- Router (Orchestrator) Agent
Every agent communicates seamlessly by passing information structured exactly based on the fashions we’ve outlined utilizing Pydantic. This clear construction ensures that every agent’s enter and output are predictable and validated, considerably decreasing runtime errors and enhancing system robustness.
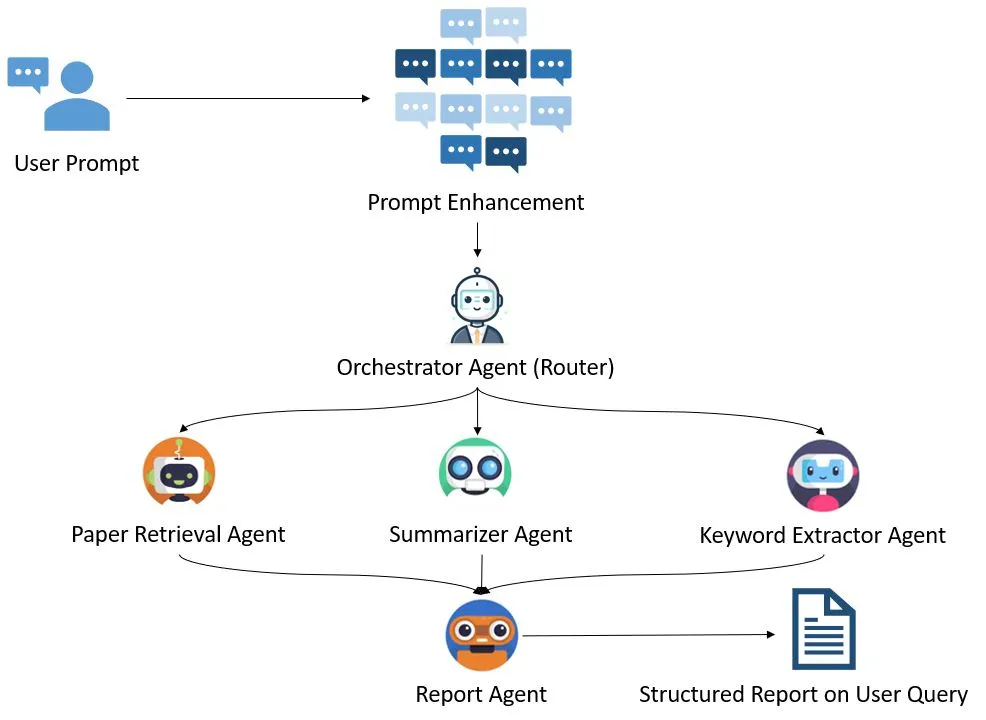
Subsequent, we’ll dive deeper into every agent, clearly explaining their implementation, position, and anticipated outputs.
Structuring the Multi-Agent Framework
With clear and validated information fashions outlined utilizing Pydantic, we now flip to the design and construction of our multi-agent framework. Every agent in our framework has a devoted accountability and interacts seamlessly with different brokers to carry out advanced duties collaboratively.
In our system, now we have outlined 5 specialised brokers, every serving a transparent and distinct position:
Immediate Processor Agent
The Immediate Processor Agent is step one within the workflow. Its major accountability is to take a person’s uncooked enter or question (comparable to “AI brokers in reinforcement studying”) and refine it right into a extra exact, structured search question. This refinement considerably improves the relevance of outcomes returned by exterior analysis databases.
Tasks:
- Receives the person’s preliminary question.
- Generates a refined and structured search question for optimum relevance.
Paper Retrieval Agent
The Paper Retrieval Agent receives the refined question from the Immediate Processor. It communicates immediately with exterior educational databases (like arXiv) to retrieve an inventory of related educational papers primarily based on the refined question.
Tasks:
- Interacts with exterior APIs (e.g., arXiv API).
- Retrieves a structured listing of papers, every represented utilizing the PaperMetadata mannequin.
Upon receiving paper abstracts, the Key phrase Extraction Agent routinely identifies and extracts probably the most related key phrases. These key phrases assist researchers shortly assess the main target and relevance of every paper.
Tasks:
- Extracts significant key phrases from abstracts.
- Facilitates fast evaluation and categorization of papers.
Summarization Agent
The Summarization Agent takes every paper’s summary and generates concise, informative summaries. Summaries present researchers with fast insights, saving substantial studying effort and time.
Tasks:
- Produces concise and clear summaries from paper abstracts.
- Permits sooner analysis of paper content material relevance.
Router Agent (Orchestrator)
The Router Agent is central to our multi-agent system. It coordinates the whole workflow, managing communication and information circulation amongst all different brokers. It initiates the Immediate Processor, passes refined queries to the Paper Retrieval Agent, and additional routes paper abstracts to the Key phrase Extraction and Summarization brokers. In the end, the Router compiles all outcomes right into a structured closing report.
Tasks:
- Coordinates interactions and information circulation between all brokers.
- Manages the asynchronous orchestration of agent workflows.
- Aggregates the outputs (key phrases, summaries, paper metadata) into structured closing reviews.
Transient Clarification of Agent Interactions
Our brokers work together in a transparent, sequential workflow:
- Immediate Processor Agent receives and refines the person question.
- The refined question is shipped to the Paper Retrieval Agent, retrieving related papers.
- For every retrieved paper, the Router Agent sends the summary concurrently to:
- As soon as key phrases and summaries are generated, the Router Agent compiles and aggregates them right into a closing structured report.
By structuring our brokers this fashion, we obtain a modular, maintainable, and extremely scalable analysis assistant system. Every agent could be individually enhanced, debugged, and even changed with out impacting the general system stability. Subsequent, we’ll dive deeper into every agent’s precise implementation particulars, together with clearly defined code snippets and anticipated outputs.
Refining Analysis Queries with the Immediate Processor Agent
When looking huge educational databases like arXiv, the standard and specificity of the question immediately affect the relevance and usefulness of returned outcomes. A obscure or broad question like “AI brokers” would possibly yield 1000’s of loosely related papers, making it difficult for researchers to determine actually precious content material. Thus, it’s essential to refine preliminary queries into exact, structured search statements.
The Immediate Processor Agent addresses this precise problem. Its major accountability is to rework the person’s normal analysis subject right into a extra particular, clearly scoped question. This refinement considerably improves the standard and precision of retrieved papers, saving researchers appreciable effort.
Beneath, we current the implementation of the Immediate Processor, leveraging primary heuristics to create structured queries:
@prompt_processor_agent.device
async def process_prompt(ctx: RunContext[ResearchContext], subject: str) -> str:
subject = subject.strip().decrease()
# Fundamental heuristic refinement
if ' in ' in subject:
# Break up the subject into key components if it incorporates 'in', to type exact queries.
subtopics = subject.break up(' in ')
main_topic = subtopics[0].strip()
context = subtopics[1].strip()
refined_query = f"all:{main_topic} AND cat:{context.exchange(' ', '_')}"
else:
# Fallback: Assume it is a broader subject
refined_query = f"ti:"{subject}" OR abs:"{subject}""
return refined_query
Clarification of the Improved Implementation
- Enter normalization: The agent begins by trimming and changing the enter subject to lowercase to make sure consistency.
- Contextual parsing: If the person’s subject consists of the key phrase “in” (for instance, “AI brokers in reinforcement studying”), the agent splits it into two clear components:
- A major subject (AI brokers)
- A particular context or subfield (reinforcement studying)
- Structured question constructing: Utilizing these parsed elements, the agent generates a exact question that explicitly searches the first subject throughout all fields (all:) and restricts the search to papers categorized or intently associated to the required context.
- Fallback dealing with: If the subject doesn’t explicitly embody contextual cues, the agent generates a structured question that searches immediately throughout the title (ti:) and summary (abs:) fields, boosting relevance for normal searches.
Anticipated Output Instance
When supplied with the person question: “AI brokers in reinforcement studying”
The Immediate Processor Agent would output the refined question as: “all:ai brokers AND cat:reinforcement_learning“
For a broader question, comparable to: “multi-agent methods”
The agent’s refined question can be: ‘ti:”multi-agent methods” OR abs:”multi-agent methods“
Whereas this implementation already considerably improves search specificity, there’s room for additional sophistication, together with:
- Pure Language Processing (NLP) methods for higher semantic understanding.
- Incorporation of synonyms and associated phrases to broaden queries intelligently.
- Leveraging a massive language mannequin (LLM) to interpret person intent and type extremely optimized queries.
These refined queries are structured to optimize search relevance and retrieve extremely focused educational papers.
Fetching Analysis Papers Effectively with the Paper Retrieval Agent
After refining our search queries for optimum relevance, the subsequent step is retrieving acceptable educational papers. The Paper Retrieval Agent serves exactly this position: it queries exterior educational databases, comparable to arXiv, to gather related analysis papers primarily based on our refined question.
By integrating seamlessly with exterior APIs like arXiv’s API, the Paper Retrieval Agent automates the cumbersome guide job of looking and filtering by huge quantities of educational literature. It makes use of structured information fashions (outlined earlier utilizing Pydantic) to make sure constant, clear, and validated information flows downstream to different brokers, like summarizers and key phrase extractors.
Beneath is a sensible instance of the Paper Retrieval Agent’s implementation:
@paper_retrieval_agent.device
async def fetch_papers(ctx: RunContext[ResearchContext]) -> listing[PaperMetadata]:
search = arxiv.Search(
question=ctx.deps.question,
max_results=5,
sort_by=arxiv.SortCriterion.SubmittedDate
)
outcomes = listing(search.outcomes())
papers = []
for end in outcomes:
published_str = (
outcome.printed.strftime("%Y-%m-%d")
if hasattr(outcome, "printed") and outcome.printed just isn't None
else "Unknown"
)
paper = PaperMetadata(
title=outcome.title,
summary=outcome.abstract,
authors=[author.name for author in result.authors],
publication_date=published_str
)
papers.append(paper)
return papers
Clarification of the Implementation
- The agent makes use of the refined question (ctx.deps.question) acquired from the Immediate Processor Agent to provoke a search by way of the arXiv API.
- It specifies max_results=5 to retrieve the 5 newest papers related to the question, sorted by their submission date.
- Every retrieved outcome from arXiv is structured explicitly right into a PaperMetadata object utilizing our beforehand outlined Pydantic mannequin. This structured strategy ensures validation and information consistency.
- The structured information is collected into an inventory and returned, prepared for consumption by downstream brokers.
Highlighting Pydantic’s Function
Utilizing Pydantic fashions to construction responses from exterior APIs gives important advantages:
- Information validation: Ensures all required fields (title, summary, authors, publication date) are at all times supplied and appropriately formatted.
- Consistency: Ensures downstream brokers obtain uniformly structured information, simplifying processing logic.
- Debugging and Upkeep: Structured schemas considerably cut back errors, enhancing maintainability and simplifying debugging.
Anticipated Output Instance
Upon executing the retrieval agent with a refined question (e.g., “all:ai brokers AND cat:reinforcement_learning”), you’d anticipate structured outputs like:
[
{
"title": "Deep Reinforcement Learning with Agentic Systems",
"abstract": "This paper discusses advancements in agentic reinforcement
learning...",
"authors": ["Alice Smith", "John Doe"],
"publication_date": "2025-03-20"
},
{
"title": "Agent Coordination in Reinforcement Studying Environments",
"summary": "We discover strategies for enhancing multi-agent coordination...",
"authors": ["Jane Miller", "Bob Johnson"],
"publication_date": "2025-03-18"
}
// (three further related structured outcomes)
]
Such structured outputs empower additional automated evaluation by subsequent brokers, enabling environment friendly key phrase extraction and summarization.
As soon as related papers have been retrieved, effectively categorizing and summarizing their content material is essential. Researchers usually want fast methods to determine the core ideas and key concepts inside a big physique of literature with out having to learn each summary intimately.
That is the place Key phrase Extraction performs a pivotal position. Mechanically extracting key phrases from abstracts helps researchers shortly decide the principle focus of every paper and determine rising developments or group-related analysis extra successfully.
The Key phrase Extraction Agent explicitly targets this want. Given a paper’s summary, it identifies a set of important phrases representing the summary’s content material.
Code Snippet (Key phrase Extraction Agent):
@keyword_extraction_agent.device
async def extract_keywords(ctx: RunContext[ResearchContext], summary: str)
-> KeywordResult:
# Fundamental key phrase extraction logic (placeholder implementation)
phrases = summary.break up()
seen = set()
unique_words = []
for phrase in phrases:
normalized = phrase.strip('.,;:"()').decrease()
if normalized and normalized not in seen:
seen.add(normalized)
unique_words.append(normalized)
if len(unique_words) >= 5:
break
return KeywordResult(key phrases=unique_words)
Clarification of the Implementation
- The agent takes the paper summary as enter.
- It splits the summary textual content into particular person phrases, normalizing them to take away punctuation and changing them to lowercase.
- It then gathers the primary 5 distinctive phrases as key phrases. This can be a simplified implementation supposed to display key phrase extraction clearly.
- Lastly, it returns a structured KeywordResult containing these extracted key phrases.
Highlighting Pydantic’s Profit
By utilizing Pydantic’s clearly outlined schema (KeywordResult), key phrase outputs stay structured and constant, making it easy for downstream brokers (just like the summarization or orchestration brokers) to eat this information with out ambiguity.
Anticipated Output Instance
Given a pattern summary:
"This paper discusses developments in agentic reinforcement studying,
specializing in deep studying methods for enhancing agent cooperation."
The Key phrase Extraction Agent would produce an output like:
["this", "paper", "discusses", "advancements"]
Be aware: This simplistic extraction logic is a placeholder demonstrating primary key phrase extraction. Precise manufacturing implementations would usually make use of extra superior Pure Language Processing (NLP) methods (comparable to TF-IDF, RAKE, or language model-based extraction) to generate key phrases of upper relevance.
Summarizing Papers Concisely with the Summarization Agent
In a tutorial analysis setting, time effectivity is crucial. Researchers usually face an amazing variety of papers and abstracts. Automated summaries permit fast scanning and identification of probably the most related analysis with out studying by whole abstracts or papers.
The Summarization Agent tackles this problem immediately. It generates concise and significant summaries from the paper abstracts, enabling researchers to quickly decide every paper’s relevance and determine whether or not deeper investigation is warranted.
Code Snippet (Summarization Agent)
@summary_agent.device
async def summarize_paper(ctx: RunContext[ResearchContext], summary: str)
-> PaperSummary:
summary_text = summary[:150] + "..." if len(summary) > 150 else summary
return PaperSummary(abstract=summary_text)
Clarification of the Implementation
- The agent accepts the paper summary as enter.
- It generates a brief abstract by extracting the primary 150 characters from the summary, appending “…” if the summary exceeds this size.
- The abstract is then returned as a structured PaperSummary object, making certain constant formatting and facilitating additional automation or reporting duties.
This easy summarization strategy gives a fast snapshot of every paper’s content material. Whereas simple, it’s efficient for preliminary assessments, enabling researchers to shortly display a number of abstracts.
Anticipated Output Instance (Textual content Solely)
Given the summary:
"This paper discusses developments in agentic reinforcement studying,
specializing in deep studying methods for enhancing agent cooperation in
multi-agent environments. We suggest novel algorithms and consider their
effectiveness by in depth simulations."
The Summarization Agent would produce:
"This paper discusses developments in agentic reinforcement studying,
specializing in deep studying methods for enhancing agent cooperation in
multi-age..."
Potential for Superior Summarization Methods
Whereas our implementation gives rapid worth, integrating superior summarization fashions—comparable to transformer-based language fashions (e.g., GPT fashions, T5, or BART)—might considerably improve abstract high quality, coherence, and contextual accuracy.
Leveraging refined summarization methods would yield extra informative and contextually exact summaries, additional enhancing researchers’ effectivity and accuracy when evaluating papers.
Now, we are able to transfer on to the ultimate and central piece of our system: The Router Agent (Orchestrator).
Bringing all of it Collectively: Agentic Orchestration
On the coronary heart of a multi-agent system lies the orchestration logic. This element ensures easy coordination and communication amongst numerous specialised brokers, managing workflows, dependencies, and the sequential or parallel execution of duties.
In our analysis assistant system, the Router Agent (Orchestrator) performs this central position. It coordinates information circulation between particular person brokers such because the Immediate Processor, Paper Retrieval, Key phrase Extraction, and Summarization brokers. Doing so ensures environment friendly dealing with of person queries, retrieval of related analysis, extraction of significant insights, and clear presentation of outcomes.
Let’s now study how the Router Agent orchestrates this whole workflow:
Code Snippet (Router Agent Orchestration)
@router_agent.device
async def orchestrate_workflow(ctx: RunContext[ResearchContext]) -> str:
print("Beginning immediate processing...")
refined_query = await prompt_processor_agent.run(ctx.deps.question,
deps=ctx.deps)
print(f"Refined Question: {refined_query.information}")
print("Fetching papers...")
papers = await paper_retrieval_agent.run(refined_query.information, deps=ctx.deps)
print(f"Fetched {len(papers.information)} papers.")
response = "Remaining Report:n"
for paper in papers.information:
print(f"nProcessing paper: {paper.title}")
print("Extracting key phrases...")
key phrases = await keyword_extraction_agent.run(paper.summary,
deps=ctx.deps)
print(f"Extracted Key phrases: {key phrases.information.key phrases}")
print("Producing abstract...")
abstract = await summary_agent.run(paper.summary, deps=ctx.deps)
print(f"Generated Abstract: {abstract.information.abstract}")
response += (
f"nTitle: {paper.title}n"
f"Key phrases: {key phrases.information.key phrases}n"
f"Abstract: {abstract.information.abstract}n"
)
return response
Step-by-step Clarification of Orchestration Logic
- Immediate Processing:
- The Router Agent first passes the preliminary person question to the Immediate Processor Agent.
- The Immediate Processor refines the question, and the Router logs the refined question clearly.
- Paper Retrieval:
- Utilizing the refined question, the Router invokes the Paper Retrieval Agent to fetch related educational papers from arXiv.
- After retrieval, it logs the variety of papers fetched, enabling visibility into the system’s exercise.
- Processing Every Paper: For every paper retrieved, the Router performs two key duties concurrently:
- Key phrase Extraction: It passes every summary to the Key phrase Extraction Agent and logs the key phrases extracted.
- Summarization: It additionally invokes the Summarization Agent for every summary, logging the concise abstract obtained.
- Aggregating Outcomes: The Router aggregates all data—titles, key phrases, summaries—right into a structured, human-readable “Remaining Report.”
- The asynchronous (async/await) nature of the orchestration permits simultaneous job execution, considerably enhancing workflow effectivity, particularly when coping with exterior API calls.
- Structured logging at every step gives clear visibility into the workflow, facilitating simpler debugging, traceability, and future upkeep or growth of the system.
With our orchestration clearly outlined, we are able to now conclude the pipeline by producing skilled, structured reviews.
Producing Skilled Outputs with Structured Information
In the end, the worth of an automatic analysis assistant lies not solely in its effectivity but additionally within the readability and professionalism of its closing outputs. Researchers usually want structured, easy-to-read paperwork that consolidate key insights clearly. Changing structured information from our multi-agent system into skilled reviews (like PDFs) enhances readability and usefulness.
With the structured information output now we have from our Router Agent, producing a cultured PDF report is simple. Right here’s how we leverage the structured information to create clear, visually interesting PDF reviews utilizing Python:
Code Snippet (PDF Technology)
def generate_pdf_report(report_text: str, output_filename: str = "Final_Report.pdf"):
import markdown2
from xhtml2pdf import pisa
# Convert the structured markdown textual content to HTML
html_text = markdown2.markdown(report_text)
# Create and save the PDF file
with open(output_filename, "w+b") as result_file:
pisa.CreatePDF(html_text, dest=result_file)
Clarification of the PDF Technology Logic
- Markdown Conversion: The structured closing report, generated by our Router Agent, is initially in a structured textual content or markdown format. We convert this markdown textual content into HTML utilizing the markdown2 library.
- PDF Technology: The xhtml2pdf library takes the transformed HTML content material and generates a professional-looking PDF file, neatly formatted for readability.
- Ease Because of Structured Information: The structured outputs from our brokers, facilitated by our Pydantic information fashions, make sure the markdown content material is persistently formatted. This consistency simplifies conversion into high-quality PDFs with out guide intervention or further parsing complexity.
Anticipated Output
Knowledgeable PDF is generated after operating the snippet with our structured report as enter. This PDF will neatly current every paper’s title, key phrases, and abstract clearly, making it straightforward for researchers to shortly evaluation, distribute, or archive their findings.
With this step, our multi-agent analysis assistant pipeline is full, successfully automating literature discovery, processing, and reporting in a structured, environment friendly, {and professional} method. Subsequent, we glance into a couple of sensible examples of the magnetic framework.
Multi-Agent System in Motion: Sensible Examples
Let’s discover how our multi-agent analysis assistant performs throughout totally different analysis eventualities. We’ll display the system’s effectiveness by presenting three distinct prompts. Every instance showcases how a easy person question transforms right into a complete, structured, and professionally formatted analysis report.
Instance 1: Reinforcement Studying Brokers
For our first situation, we discover latest analysis on making use of reinforcement studying to robotics.
Consumer Immediate:
"Reinforcement studying brokers in robotics"
Beneath is a screenshot of the multi-agent workflow output, clearly illustrating how the immediate was refined, related papers retrieved, key phrases extracted, and summaries generated.
Beginning immediate processing...
Refined Question: all:reinforcement studying brokers AND cat: robotics
Fetching papers...
<ipython-input-5-08d1ccafd1dc>:46: DeprecationWarning: The 'Search.outcomes' technique
is deprecated, use 'Shopper.outcomes' as an alternative
outcomes
Fetched
listing(search.outcomes())
papers.
Beginning immediate processing...
Refined Question: all: ("reinforcement studying brokers" OR "reinforcement studying" OR
"RL brokers") AND cat: robotics Fetching papers...
Fetched
papers.
Beginning immediate processing... Beginning immediate processing.... Beginning immediate
processing.... Beginning immediate processing...
Beginning immediate processing...
Refined Question: all: ("reinforcement studying brokers" OR "RL brokers") AND cat:
robotics
Fetching papers...
Refined Question: ti:"reinforcement studying brokers robotics" OR abs: "reinforcement
studying brokers robotics"
Fetching papers...
Refined Question: all: ("reinforcement studying brokers" OR "reinforcement studying" OR
"RL brokers") AND cat: robotics
Fetching papers...
Refined Question: all: ("reinforcement studying brokers" OR "RL brokers") AND cat:
robotics
Fetching papers...
Refined Question: ti: "reinforcement studying brokers" OR ti:"reinforcement studying" OR
ti: "RL brokers" OR abs: "reinforcement studying brokers" OR abs: "reinforcement
studying" OR abs: "RL brokers" AND cat: robotics Fetching papers...
Discover above how the person immediate is being refined iteratively for higher search capabilities.
Fetched 1 papers.
Processing paper: An Structure for Unattended Containerized (Deep) Reinforcement
Studying with Webots Extracting key phrases...
Extracted Key phrases: ['data science', 'reinforcement learning', '3D worlds',
'simulation software', 'Robotino', 'model development', 'unattended training', 'Webots', 'Robot Operating System', 'APIs', 'container technology', 'robot tasks']
Producing abstract... Abstract: This paper critiques instruments and approaches for coaching
reinforcement studying brokers in 3D environments, particularly for the Robotino robotic. It addresses the problem of separating the simulation setting from the
mannequin improvement envi Beginning immediate processing... Refined Question: ti:
"reinforcement studying brokers for robotics" OR abs: "reinforcement studying brokers
for robotics"
Fetching papers...
Fetched 1 papers.
Processing paper: An Structure for Unattended Containerized (Deep) Reinforcement
Studying with Webots Extracting key phrases...
Extracted Key phrases: ['data science', 'reinforcement learning', '3D simulation',
'Robotino', 'simulation software', 'Webots', 'Robot Operating System', 'unattended
training pipelines', 'APIS', 'model development', 'container technology', 'virtual
wo Generating summary... Summary: This paper reviews tools and approaches for
training reinforcement learning agents in 3D worlds, focusing on the Robotino
robot. It highlights the challenge of integrating simulation environments for
virtual world creators and model develo Final Report:
### Comprehensive Report on "Reinforcement Learning Agents for Robotics"
#### Title:
An Architecture for Unattended Containerized (Deep) Reinforcement Learning with
Webots
#### Authors:
Tobias Haubold, Petra Linke
#### Publication Date: February 6, 2024 #### Abstract:
As data science applications gain traction across various industries, the tooling
landscape is evolving to support the lifecycle of these applications, addressing
challenges to enhance productivity. In this context, reinforcement learning (RL)
for This paper reviews various tools and strategies for training reinforcement
learning agents specifically for robotic applications in 3D spaces, utilizing the
Robotino robot. It examines the critical issue of separating the simulation
environment for The authors propose a solution that isolates data scientists from
the complexities of simulation software by using Webots for simulation, the Robot
Operating System (ROS) for robot communication, and container technology to create
a clear division #### Keywords:
Data Science
Reinforcement Learning
- 3D Worlds
- Simulation Software
Robotino
Model Development
The multi-agent system draws and collates the information from arxiv into a single report.
You can download the complete structured PDF report below:
Download
Example 2: Quantum Machine Learning
In the second scenario, we investigate current developments in quantum machine learning.
User Prompt:
"Quantum machine learning techniques"
The following screenshot demonstrates how the system refined the query, retrieved relevant papers, performed keyword extraction, and provided concise summaries.
Starting prompt processing...
Refined Query: ti: "quantum machine learning techniques" OR abs: "quantum machine
learning techniques"
Fetching papers...
<ipython-input-5-08d1ccafd1dc>:46: DeprecationWarning: The 'Search.results' method
is deprecated, use 'Client.results' instead
results list (search.results())
Fetched 5 papers.
Processing paper: Experimental demonstration of enhanced quantum tomography via
quantum reservoir processing Extracting keywords...
Extracted Keywords: ['quantum machine learning', 'quantum reservoir processing',
'continuous-variable state reconstruction', 'bosonic circuit quantum
electrodynamics', 'measurement outcomes', 'reconstruction Generating summary...
Summary: This paper presents an experimental demonstration of quantum reservoir
processing for continuous-variable state reconstruction using bosonic quantum
circuits. It shows that the method efficiently lea Processing paper: Detection
states of ions in a Paul trap via conventional and quantum machine learning
algorithms Extracting keywords...
Extracted Keywords: ['trapped ions', 'quantum technologies', 'quantum computing'
, 'state detection', 'high-fidelity readouts', 'machine learning', 'convolution',
'support vector machine', 'quantum annealing', Generating summary... Summary: This
work develops and benchmarks methods for detecting quantum states of trapped
ytterbium ions using images from a sensitive camera and machine learning
techniques. By applying conventional and qua Processing paper: Satellite image
classification with neural quantum kernels Extracting keywords...
Extracted Keywords: ['quantum machine learning', 'satellite image classification',
'earth observation', 'solar panels', 'neural quantum kernels', 'quantum neural networks', 'classical pre-processing', 'dimens Generating summary... Summary: This
paper presents a novel quantum machine learning approach for classifying satellite
images, particularly those with solar panels, relevant to earth observation. It
combines classical pre-processi Processing paper: Harnessing Quantum Extreme
Learning Machines for image classification
Extracting keywords...
Extracted Keywords: ['quantum machine learning', 'image classification', 'quantum
extreme learning machine', 'quantum reservoir', 'feature map', 'dataset
preparation', 'Principal Component Analysis', 'Auto-En Generating summary...
Summary: This research explores quantum machine learning techniques for image
classification, focusing on a quantum extreme learning machine that utilizes a
quantum reservoir. It analyzes various encoding met Processing paper: Quantum
Generative Adversarial Networks: Generating and Detecting Quantum Product States
Extracting keywords...
Extracted Keywords: ['quantum machine learning', 'QGAN', 'quantum product states',
'image generation', 'decoherence', 'NISQ devices', 'GAN MinMax', 'quantum style
parameters', 'generator', 'discriminator'] Producing abstract... Abstract: The paper
introduces a Quantum Generative Adversarial Community (OGAN), leveraging quantum
machine studying to generate and discriminate quantum product states, a job with
no classical analog. It util
Obtain the complete PDF report for detailed insights:
Obtain Quantum_Machine_Learning_Report.pdf
Instance 3: AI Brokers
For our third situation, we study how multi-agent methods are being utilized .
Consumer Immediate:
"Multi-agent methods"
Beneath, you may clearly see the screenshot of our system’s output, which exhibits structured question refinement, retrieval of pertinent analysis papers, key phrase extraction, and summarization.
Beginning immediate processing...
Refined Question: ti:"ai brokers" OR abs: "ai brokers"
Fetching papers...
<ipython-input-5-08d1ccafd1dc>:46: DeprecationWarning: The 'Search.outcomes' technique
is deprecated, use 'Shopper.outcomes' as an alternative
outcomes listing (search.outcomes())
Fetched 5 papers.
Processing paper: Verbal Course of Supervision Elicits Higher Coding Brokers Extracting
key phrases...
Extracted Key phrases: ['large language models', 'AI agents', 'code generation',
'software engineering', 'CURA', 'code understanding', 'reasoning agent', 'verbal
process supervision', 'benchmark improvement', 'BigC Generating summary...
Summary: This work introduces CURA, a code understanding and reasoning agent system
enhanced with verbal process supervision (VPS), which achieves a 3.65% improvement
on challenging benchmarks. When combined wit
Processing paper: How to Capture and Study Conversations Between Research
Participants and ChatGPT: GPT for Researchers (g4r.org) Extracting keywords...
Extracted Keywords: ['large language models', 'LLMs', 'GPT for Researchers', 'G4R',
'AI systems', 'human-AI communication', 'consumer interactions', 'AI-assisted
decision-making', 'GPT Interface', 'research tool Generating summary... Summary: The
paper introduces GPT for Researchers (G4R), a free online platform designed to aid
researchers in studying interactions with large language models (LLMs) like
ChatGPT. G4R allows researchers to enab Processing paper: Collaborating with AI
Agents: Field Experiments on Teamwork, Productivity, and Performance Extracting
keywords...
Extracted Keywords: ['AI agents', 'productivity', 'performance', 'work processes',
'MindMeld', 'experimentation platform', 'human-AI teams', 'communication', 'collaboration', 'multimodal workflows', 'AI personal Generating summary... Summary:
This study introduces MindMeld, a platform for human-AI collaboration, showing that
AI agents can significantly improve productivity and performance in team settings.
In an experiment with 2310 particip Processing paper: Metacognition in Content-
Centric Computational Cognitive C4 Modeling Extracting keywords...
Extracted Keywords: ['AI agents', 'human behavior', 'metacognition', 'C4 modeling',
'cognitive robotic applications', 'neuro symbolic processing', 'LEIA Lab',
'cognitive capabilities', 'information storage', 'LL Generating summary... Summary:
This paper discusses the necessity of metacognition for AI agents to replicate
human behavior through effective information processing. It introduces content-
centric computational cognitive (C4) modelin Processing paper: OvercookedV2:
Rethinking Overcooked for Zero-Shot Coordination
Extracting keywords...
Extracted Keywords: ['AI agents', 'zero-shot coordination (ZSC)', 'Overcooked',
'state augmentation', 'coordination capabilities', 'out-of-distribution challenge',
'OvercookedV2', 'asymmetric information', 'stoc Generating summary... Summary: This
paper explores the challenges of zero-shot coordination (ZSC) in AI agents using
the Overcooked environment. It introduces a state augmentation mechanism to improve
training by incorporating states Starting prompt processing...
Refined Query: ti: "ai agents" OR abs: "ai agents"
Fetching papers...
Fetched 5 papers.
Processing paper: Verbal Process Supervision Elicits Better Coding Agents
Extracting keywords...
You can download the professionally formatted PDF report from the link below:
Download Multi_Agent_Systems_Report.pdf
Each example clearly illustrates our multi-agent framework’s ability to swiftly and effectively automate research workflows—from refining initial queries to generating structured, professional reports, all leveraging the structured data validation power of Pydantic.
Conclusion
In this blog, we’ve explored the design and implementation of a structured, scalable, and efficient Multi-Agent Research Assistant System using Pydantic. By clearly defining structured data models, we’ve ensured consistency and reliability across interactions between multiple intelligent agents—ranging from refining user prompts, retrieving relevant academic papers, extracting meaningful keywords, and summarizing complex abstracts, to orchestrating the entire workflow seamlessly. Through practical examples, we’ve demonstrated how this robust framework automates and significantly simplifies complex academic research tasks, culminating in professional-quality, ready-to-use reports.
Key Takeaways
- Pydantic ensures structured data handling, significantly reducing errors and simplifying agent interactions.
- Clear agent roles and responsibilities make multi-agent systems modular, maintainable, and scalable.
- Refined and structured queries dramatically enhance the relevance and usefulness of retrieved research.
- Automated keyword extraction and summarization save researchers valuable time, enabling rapid content assessment.
- Effective orchestration with structured logging and asynchronous workflows enhances system efficiency and ease of debugging.
By adopting such a structured multi-agent approach, developers and researchers can significantly enhance productivity, clarity, and efficiency in their research automation pipelines.
Bonus: While it was challenging to include the detailed outputs for each code block to maintain the scope of the blog, the entire code for the agentic system discussed here is being open-sourced to allow better learning and usability for the readers! (Code)
Frequently Asked Questions
A. Pydantic provides powerful runtime data validation and clearly defined schemas, ensuring reliable communication and consistency among different agents in a multi-agent setup.
A. Yes, the modular design allows seamless integration with various external APIs or databases—simply by defining appropriate agents and using structured data models.
A. The provided keyword extraction and summarization implementations are simplified for demonstration purposes. For production use, more advanced NLP techniques or fine-tuned language models are recommended to improve accuracy and relevance.
A. Leveraging asynchronous programming and structured logging (as shown in this blog) greatly improves agent efficiency. Additionally, deploying your agents in distributed or cloud environments can further enhance scalability and responsiveness.
A. Absolutely! Because the data is structured using Pydantic models, the final report generation can easily be adapted to various formats like Markdown, HTML, PDF, or even interactive dashboards.
The media shown in this article is not owned by Analytics Vidhya and is used at the Author’s discretion.
Login to continue reading and enjoy expert-curated content.