For each Python programmer, whether or not within the area of information science and machine studying or software program growth, Python slicing operations are one of the vital environment friendly, versatile, and highly effective operations. Python slicing syntax permits the extraction and modification of information constructions like Lists, Strings, Tuples, Arrays, Pandas DataFrames, and even byte sequences. Whether or not we now have to extract a bit of listing slicing in Python, manipulate characters utilizing string slicing, or simply need to streamline the workflow, slicing affords a concise technique to work with information with out the usage of advanced loops or handbook indexing. On this Python slicing tutorial, we are going to dive deeper into how the Python slicing operations work and learn to use them successfully in applications and workflows.
What Are Slicing Operations in Python?
Slicing means slicing. Equally, in Python, it means accessing or extracting a sub-sequence (portion) of a sequence (like strings, lists, tuples, or arrays) by specifying a spread of indices. Slicing operations in Python contain utilizing colon operators [:] throughout the sq. brackets. The essential syntax entails:
[START: END: STEP]
START: Begin is the index from the place the slicing begins.
END: Finish is the index level as much as which the operation shall be carried out, i.e., it’s not included within the
operation.
STEP: Step is the increment index. Its default worth is 1, which implies the entire sequence would be the output. If step=2, then each alternate worth shall be printed.
Why Use Slicing?
Slicing is a crucial technique because it permits us to entry and manipulate the information concisely, making the code extra readable and versatile throughout information constructions. For instance:
Iterating with out Slicing
lst = [1, 2, 3, 4, 5]
sublist = []
for i in vary(1, 4):
sublist.append(lst[i])
print(sublist)
Iterating with Slicing
lst = [1, 2, 3, 4, 5]
sublist = lst[1:4]
print(sublist)
Output: [2, 3, 4]
Slicing Strategies in Python
Principally, Python affords 2 other ways of slicing. One is [start: end: step] and the opposite is the .slice(begin, cease, step) operate. On this part, first we are going to undergo the syntax of those slicing operations, after this we are going to discover the main varieties of slicing that we are able to carry out in Python.
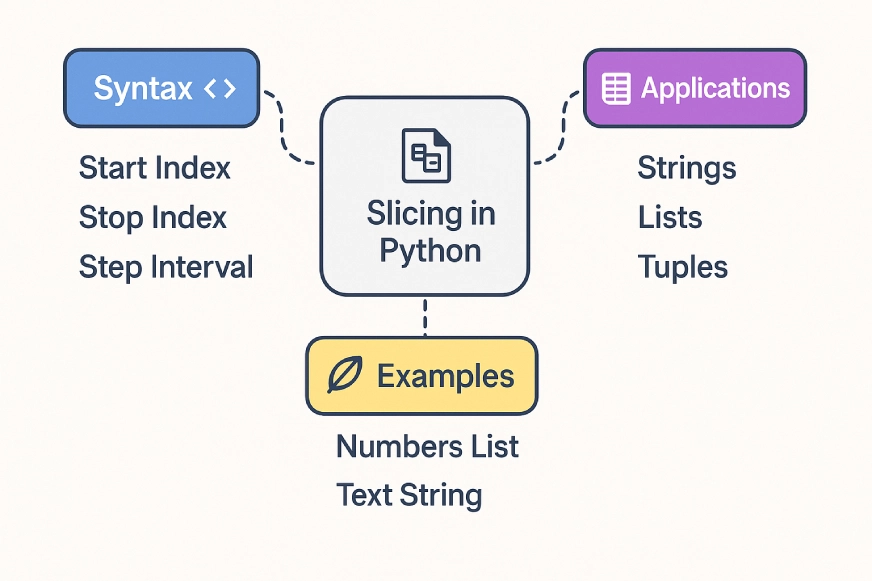
- Utilizing [start: end: step]
That is the most typical technique to carry out the slicing operation on totally different elements of the enter sequence.
Slicing with index [:] Examples
mixed_data = [10, "apple", 3.14, "banana", 42, "cherry"]
# Slice from index 1 to 4
print(mixed_data[1:4])
print(20*"--")
# Slice the listing from the begin to index 3
print(mixed_data[:3])
print(20*"--")
# Slice each 2nd component
print(mixed_data[::2])
![Slicing with index [:] Examples](https://cdn.analyticsvidhya.com/wp-content/uploads/2025/05/img_2.webp)
- Utilizing Python slice() operate
The slice operate permits you to create a slice object, which shall be utilized to the sequence. This helps whenever you need to retailer the slice specs and apply them to a number of sequences.
Syntax of .slice()
slice(begin, cease, step)
begin: The beginning index of the slice (inclusive).
cease: The stopping index of the slice (unique).
step: The step or stride (how a lot to increment the index after every step, elective).
Slicing with slice(). Examples
textual content = "Hey, world!"
# Create a slice object to get the primary 5 characters
s = slice(0, 5)
# Apply the slice object to the string
print(textual content[s])
# Output: "Hey"
Slicing each third component
mixed_data = [10, "apple", 3.14, "banana", 42, "cherry"]
s = slice(None, None, 3)
# Apply the slice object to the listing
print(mixed_data[s])
# Output: [10, 'banana']
Slice from index 2 to the top
s = slice(2, None)
# Apply the slice object to the listing
print(mixed_data[s])
# Output: [3.14, 'banana', 42, 'cherry']
Slice in reverse order
s = slice(None, None, -1)
# Apply the slice object to the listing
print(mixed_data[s])
# Output: ['cherry', 42, 'banana', 3.14, 'apple', 10]
Now we are going to look into the main varieties of slicing operations in Python
1. Fundamental Slicing
Fundamental slicing refers to extracting a subsequence for information varieties like string, listing, or tuple utilizing syntax [start: end: step]. It’s a basic software in Python that permits us to retrieve the subsequences simply. It additionally works with quite a lot of information varieties, making it a flexible approach.
numbers = [10, 20, 30, 40, 50, 60]
# Slice from index 1 to index 4 (unique)
print(numbers[1:4])
# Output: [20, 30, 40]
textual content = "Hey, world!"
# Slice from index 7 to index 12 (unique)
print(textual content[7:12])
# Output: "world"
numbers_tuple = (1, 2, 3, 4, 5, 6)
# Slice from index 2 to index 5 (unique)
print(numbers_tuple[2:5])
# Output: (3, 4, 5)
2. Omitting ‘begin’, ‘cease’, or ‘step’
Omitting begin, cease, and step in slicing permits customers to make use of default values.
- Omitting begin defaults to the start of the sequence.
- Omitting cease means the slice goes till the top.
- Omitting step defaults to a step of 1.
Eradicating these elements makes the code extra concise and versatile. It allows you to create dynamic and generalized slicing with out explicitly defining the entire parameters.
Modifying Lists with Slicing
numbers = [10, 20, 30, 40, 50, 60]
# Omitting begin, slice from the start to index 4 (unique)
print(numbers[:4])
# Output: [10, 20, 30, 40]
# Omitting cease, slice from index 2 to the top
print(numbers[2:])
# Output: [30, 40, 50, 60]
Empty Slicing
numbers_tuple = (1, 2, 3, 4, 5, 6)
# Omitting begin and step, slice the entire tuple
print(numbers_tuple[:])
# Output: (1, 2, 3, 4, 5, 6)
Delete Parts
numbers = [2,4,5,12,64,45]
numbers[1:4] = []
print(numbers)
# Output: [2,64,45]
3. Damaging Slicing
Damaging indexing permits counting from the top of a sequence. In unfavourable indexing, -1 refers back to the final component, and -2 refers back to the second final. It helps when it’s essential entry parts from the top of a sequence.
Accessing the Final Parts
numbers = [10, 20, 30, 40, 50, 60]
# Slice on the final index
print(numbers[-1])
# Output: [60]
Reversing a String
original_string = "hi there"
reversed_string = original_string[::-1]
print(reversed_string)
# Output: "olleh"
4. Slicing Utilizing Step
The step parameter permits for specifying the interval between the weather, making it helpful when processing or sampling information. A unfavourable step can reverse the sequence simply, as seen above, making it very straightforward and handy to reverse the entire information.
Slicing Each 2nd Component
numbers = [10, 20, 30, 40, 50, 60]
# Slice with step 2, selecting each second component
print(numbers[::2])
# Output: [10, 30, 50]
Complicated Step Conduct
numbers = [10, 20, 30, 40, 50, 60]
# Slice finally index
print(numbers[::-3])
# Output: [60,30]
5. Slicing with None
In Slicing, None can be utilized to symbolize the default worth for begin, cease, and finish. Utilizing None permits extra flexibility and readability within the programming. It’s a technique to apply default slicing behaviour with out defining them manually.
Omitting utilizing None
numbers = [10, 20, 30, 40, 50, 60]
# Slice each 2nd component utilizing slice(None, None, 2)
s = slice(None, None, 2)
print(numbers[s])
# Output: [10, 30, 50]
6. Out-Of-Sure Slicing
While you attempt to slice a sequence past its bounds (both with massive indices or with -ve indices out of vary), it gained’t increase any error in Python and easily return the biggest legitimate slice with out worrying in regards to the exceptions.
numbers = [10, 20, 30, 40, 50, 60]
# Slice past the size of the listing
print(numbers[4:15])
# Output: [50, 50]
Slicing Past Size
textual content = "Hey, world!"
# Slice past the size
print(textual content[15:55])
# Output: no output
7. NumPy Array Slicing
In NumPy, slicing additionally works equally to the Python fundamental slicing. Additionally, NumPy is particularly designed for scientific computing and in addition permits quicker information manipulation. This aids in additional supporting extra superior and environment friendly operations for big datasets. Slicing allows NumPy to entry sub-arrays and in addition modify them effectively (i.e., permitting us to switch the subarrays).
Slicing via 1-D Arrays
import numpy as np
# Create a 1-D NumPy array
arr = np.array([10, 20, 30, 40, 50, 60])
# Slice from index 1 to index 4 (unique)
print(arr[1:4])
# Output: [20 30 40]
Like the essential slicing, it permits us to slice via the array from index 1 to 4 (unique), identical to the common Python Slicing. It additionally permits to carry out all the opposite operations mentioned above, in arrays as effectively.
# Slice each second component from the array
print(arr[::2])
# Output: [10 30 50]
Slicing via Multi-dimensional Arrays
# Create a 2-D NumPy array (matrix)
arr_2d = np.array([[10, 20, 30], [40, 50, 60], [70, 80, 90]])
# Slice from row 1 to row 2 (unique), and columns 1 to 2 (unique)
print(arr_2d[1:2, 1:3])
# Output: [[50 60]]
8. Pandas DataFrame Slicing
Pandas DataFrames are 2-dimensional labelled information constructions that additionally assist slicing operations. It permits slicing via the information factors via .loc() and .iloc(). Together with this, Pandas additionally helps boolean indexing.
Slicing the dataframe itself permits for filtering and manipulating massive datasets effectively. It permits to pick subsets of information utilizing situations, making it a precious software for information evaluation and machine studying.
Slicing utilizing Row Index (.iloc)
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({
'A': [1, 2, 3, 4, 5],
'B': [10, 20, 30, 40, 50]
})
print(df)
# Output
# A B
# 0 1 10
# 1 2 20
# 2 3 30
# 3 4 40
# 4 5 50
# Slice the primary three rows (unique of the fourth row)
print(df.iloc[:3])
# A B
# 0 1 10
# 1 2 20
# 2 3 30
Right here, the .iloc(3) slices the primary 3 rows(index 0 to 2) of the DataFrame.
Slicing utilizing Column Identify (.loc)
# Create a DataFrame
df = pd.DataFrame({
'A': [1, 2, 3, 4, 5],
'B': [10, 20, 30, 40, 50]
})
print(df)
# Output
# A B
# 0 1 10
# 1 2 20
# 2 3 30
# 3 4 40
# 4 5 50
print(df.loc[df['A'] > 2])
# Output:
# A B
# 2 3 30
# 3 4 40
# 4 5 50
The .loc permits slicing the labels with column names or as an index as False. Right here, we’re slicing primarily based on the situation that the column ”A” worth should be higher than 2.
9. Byte Sequence Slicing
Python affords byte sequences comparable to bytes and bytearray, which assist slicing identical to lists, strings, or arrays. Byte sequence comes into the image once we are utilizing the binary information varieties, and slicing permits you to extract related elements of binary information with ease and effectivity.
Slicing a byte Object
byte_seq = b'Hey, world!'
# Slice from index 0 to index 5 (unique)
print(kind(byte_seq))
print(byte_seq[:5])
# Output: <class 'bytes'>, b'Hey'
Slicing a bytearray(Mutable bytes)
byte_arr = bytearray([10, 20, 30, 40, 50, 60])
# Slice from index 2 to index 5 (unique)
print(byte_arr[2:5])
# Output: bytearray(b'2x1e<')
Right here, the output is in values similar to ASCII characters. This occurs when the output just isn’t within the printable vary. So, bytearray(b’2x1e<‘) is the output as a result of it represents these byte values in a extra human-readable kind.
print(listing(byte_arr[2:5])) # Output: [30, 40, 50]
# Ouput: [30,40,50]
Advantages of Utilizing Slicing in Python
There are lots of advantages of utilizing slicing operations in Python, together with:
- Effectivity: Slicing permits quick entry of the specified sub-sequence out of bigger datasets while not having to loop.
- Conciseness: It allows extra concise and readable code for information manipulation.
- Flexibility: With the assistance of libraries like NumPy and Pandas, slicing via multidimensional information provides an environment friendly approach for information preprocessing.
- Reminiscence Environment friendly: Slicing is optimized for efficiency. Additionally, Python’s inside mechanism ensures that slicing operations are quick and reminiscence environment friendly, in contrast to handbook indexing, which takes a variety of handbook coding and will increase reminiscence utilization.
Issues to Keep away from Whereas Utilizing Slicing Operations
Right here are some things to keep away from whereas utilizing slicing operations in Python.
- Exceeding Index Boundaries: Slicing past the sequence size is not going to trigger any error in Python. Nonetheless, this can lead to undesirable outcomes, particularly when working with bigger datasets.
- Complicated Indexing and Slicing Syntax: The slicing syntax entails sequence[start : stop: end], however deciding on the index/place of the component we wish can even give the specified component.
- Slicing With out Contemplating Mutability: Slicing can be utilized to switch the sequence, however to make use of this, one should think about whether or not the information kind they’re coping with helps mutability or not. For instance, lists and byte arrays are mutable; then again, strings, tuples, and bytes are immutable. To allow them to’t be modified instantly via slicing.
- Slicing with Invalid Step Values: Whereas utilizing slicing, one should additionally think about the step as it can determine what number of factors shall be skipped in between. However the usage of an invalid worth can result in sudden outcomes, inflicting inefficient operations.
Conclusion
Slicing in Python is an environment friendly and highly effective approach that permits you to effectively entry and manipulate the Python information varieties like Lists, strings, tuples, NumPy arrays, and Pandas DataFrames. So, whether or not you’re slicing a listing, working with multi-dimensional arrays in NumPy, or coping with massive datasets utilizing Pandas, slicing at all times offers a transparent and concise technique to work with sequences. By mastering slicing, one can write cleaner and extra environment friendly code, which is crucial for each Python programmer.
Login to proceed studying and revel in expert-curated content material.