Crafting the right resume is a tedious activity – whether or not you’re a recent graduate moving into the job market, or a seasoned skilled aiming for that subsequent huge profession transfer. However what for those who may have a private resume reviewer at your fingertips – with out spending any cash on LinkedIn Premium or hiring an expensive skilled? Enter the world of AI-powered options for resume optimisation! With the facility of Giant Language Fashions (LLMs) and revolutionary libraries like CrewAI, now you can construct your very personal resume evaluation agent. On this weblog, I’ll present you how you can construct an agentic system with CrewAI for reviewing and optimizing resumes.
Construction of the Resume Reviewer Agentic System
Earlier than moving into the coding half, you will need to perceive how you can construction an AI system for resume optimisation. The easiest way to do that can be by noting down the duties that we want the agent to carry out. So, I’m assuming you’ve gotten already made a resume (else you possibly can obtain one resume from right here). Now, we’d need our resume evaluation agentic system to carry out 3 duties:
- Learn by way of and supply suggestions on the resume.
- Enhance or re-write the resume based mostly on the suggestions.
- Recommend acceptable jobs based mostly on the improved resume and specified location.
Now that now we have our necessities clearly written, we are able to determine on the variety of brokers and their duties. Ideally, it is suggested we go along with one activity per agent to keep away from overburdening any agent. This interprets to us constructing 3 brokers for our CrewAI-based resume evaluation agent system:
- The primary agent will present suggestions on the resume.
- The second agent will enhance the resume based mostly on the suggestions.
- And, the third agent would recommend acceptable jobs based mostly on the improved resume and specified location.
Now that you just perceive how we are able to use AI for resume optimisation, let’s leap to the Python code and construct our resume reviewer agent.
Python Code to Construct Resume Reviewer Agentic System with CrewAI
Listed here are the step-by-step directions to construct a resume evaluation agent with CrewAI. To raised comply with these steps, I recommend you watch this hands-on video parallelly.
So let’s start!
Step 1: Set up and Import Related Libraries
We’ll start with putting in the PyMuPDF, python-docx and most significantly CrewAI.
- The PyMuPDF library is used for studying PDF paperwork.
- The python-docx library is used for creating, studying, and modifying Microsoft Phrase (.docx) paperwork.
- Additionally, guarantee CrewAI is put in in your system. It is likely one of the hottest agentic frameworks to construct multi-agent programs.
#!pip set up PyMuPDF
#!pip set up python-docx
#!pip set up crewai crewai-tools
Step 2: Loading the Resume
The following step is to import the fitz and docx modules. Right here ‘fitz’ is the title you utilize to import PyMuPDF, and ‘docx’ is to import the python-docx library.
import fitz # PyMuPDF for PDF processing
import docx # python-docx for DOCX processing
We’ll now outline the next three capabilities to allow our agentic system to extract textual content from resumes saved in numerous codecs.
- The primary perform – “extract_text_from_pdf” will extract contents from the resume in PDF format.
- The second perform – “extract_text_from_docx” will extract contents from the resume in docx format.
- The third perform – “extract_text_from_resume” wraps the primary two capabilities and makes use of the respective perform based mostly on whether or not the doc is a PDF or docx.
Let’s run this.
def extract_text_from_pdf(file_path):
"""Extracts textual content from a PDF file utilizing PyMuPDF."""
doc = fitz.open(file_path)
textual content = ""
for web page in doc:
textual content += web page.get_text()
return textual content
def extract_text_from_docx(file_path):
"""Extracts textual content from a DOCX file utilizing python-docx."""
doc = docx.Doc(file_path)
fullText = []
for para in doc.paragraphs:
fullText.append(para.textual content)
return "n".be part of(fullText)
def extract_text_from_resume(file_path):
"""Determines file kind and extracts textual content."""
if file_path.endswith(".pdf"):
return extract_text_from_pdf(file_path)
elif file_path.endswith(".docx"):
return extract_text_from_docx(file_path)
else:
return "Unsupported file format."
Subsequent, let’s check this perform with 2 resumes. The primary one will likely be a resume in PDF format of an individual named Bruce Wayne (not the superhero).
res1 = extract_text_from_resume('/Customers/admin/Desktop/YT Lengthy/Bruce Wayne.pdf')
print(res1)
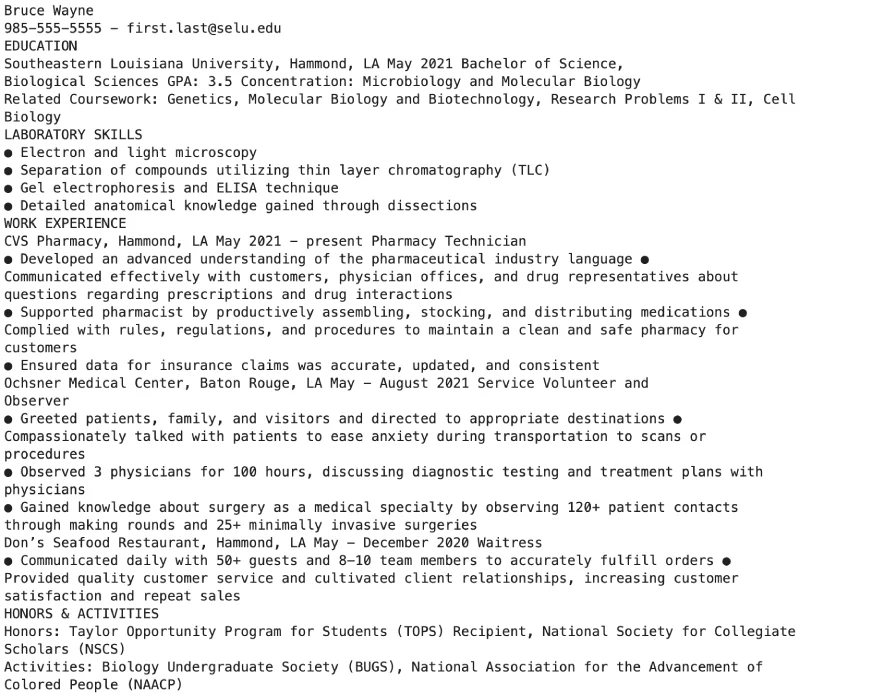
Now, we view the second resume.
res2 = extract_text_from_resume('/Customers/admin/Desktop/YT Lengthy/Anita Sanjok.docx')
print(res2)
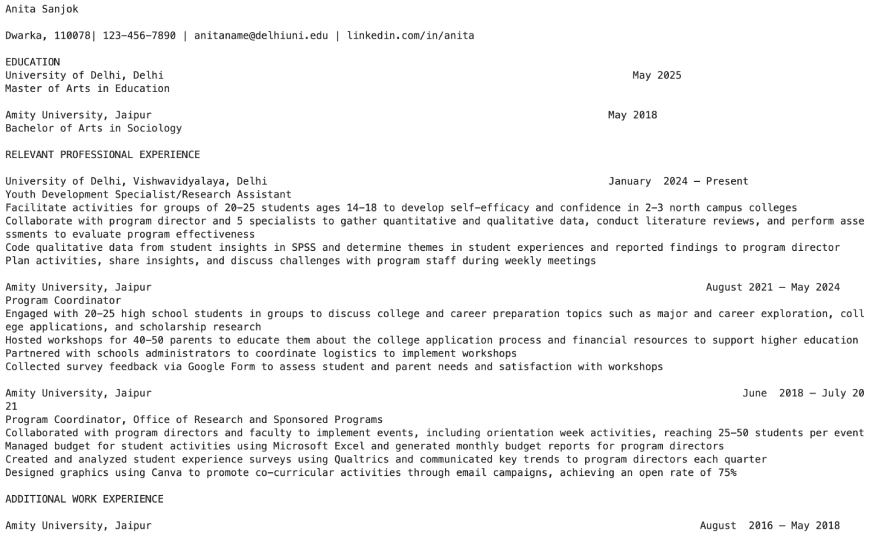
Step 3: Getting ready the Brokers and Duties
This step is the place CrewAI enters the scene. We’ll now import crewai and begin constructing the agentic system. For this, we’ll want 3 parts from CrewAI, namely- Agent, Activity and Crew.
- Agent represents an AI assistant with a selected function and purpose.
- Activity defines an goal that the agent wants to perform.
- And eventually, Crew is used to bundle a number of brokers and their respective duties collectively to work and obtain the set goal.
import os
from crewai import Agent, Activity, Crew
Subsequent, we have to add the OpenAI API key, which I’ve saved in a separate file. So, we have to learn the API key from the file and set it as an surroundings variable. On this case we’re utilizing GPT-4o-mini because the LLM.
with open('/Customers/apoorv/Desktop/AV/Code/GAI/keys/openai.txt', 'r') as file:
openai_key = file.learn()
os.environ['OPENAI_API_KEY'] = openai_key
os.environ["OPENAI_MODEL_NAME"] = 'gpt-4o-mini'
Subsequent, we outline the brokers and their respective duties.
Our first agent would be the “resume_feedback” agent. The agent class has some parameters which assist us outline the target of the agent. Lets take a look at them:
- The function parameter defines the agent’s perform and experience throughout the crew. Right here the agent’s function is that of a “Skilled Resume Advisor”.
- The purpose is the only goal that guides brokers’ decision-making.
- Verbose = True allows detailed execution logs for debugging.
- The backstory provides an in depth description of the traits of the agent. It’s much like defining the context and persona of the agent.
Let’s run this.
# Agent 1: Resume Strategist
resume_feedback = Agent(
function="Skilled Resume Advisor",
purpose="Give suggestions on the resume to make it stand out within the job market.",
verbose=True,
backstory="With a strategic thoughts and a mind for element, you excel at offering suggestions on resumes to spotlight essentially the most related abilities and experiences."
)
Now let’s outline the duty for the resume_feedback agent. The duty is nothing however the particular task that must be accomplished by the agent to which this activity is assigned.
- We’ll add an in depth description which needs to be a transparent, and concise assertion of what the duty entails. You need to notice that the {resume} written in curly braces is a placeholder that will likely be dynamically changed with an precise resume enter when the duty runs. You’ll be able to pause studying right here, undergo the outline and make modifications as per your necessities.
- The anticipated output parameter helps us decide the format of the output. You will get the output in numerous codecs resembling json, markdown or bullet factors
- The agent parameter highlights the title of the agent to which this activity is assigned.
# Activity for Resume Strategist Agent: Align Resume with Job Necessities
resume_feedback_task = Activity(
description=(
"""Give suggestions on the resume to make it stand out for recruiters.
Assessment each part, inlcuding the abstract, work expertise, abilities, and schooling. Recommend so as to add related sections if they're lacking.
Additionally give an general rating to the resume out of 10. That is the resume: {resume}"""
),
expected_output="The general rating of the resume adopted by the suggestions in bullet factors.",
agent=resume_feedback
)
We’ll do the identical for the following agent, that’s, the resume_advisor agent which writes a resume incorporating the suggestions from the resume_feedback agent and defines its activity within the resume_advisor_task. Be happy to undergo it.
# Agent 2: Resume Strategist
resume_advisor = Agent(
function="Skilled Resume Author",
purpose="Primarily based on the suggestions recieved from Resume Advisor, make modifications to the resume to make it stand out within the job market.",
verbose=True,
backstory="With a strategic thoughts and a mind for element, you excel at refining resumes based mostly on the suggestions to spotlight essentially the most related abilities and experiences."
)
# Activity for Resume Strategist Agent: Align Resume with Job Necessities
resume_advisor_task = Activity(
description=(
"""Rewrite the resume based mostly on the suggestions to make it stand out for recruiters. You'll be able to regulate and improve the resume however do not make up details.
Assessment and replace each part, together with the abstract, work expertise, abilities, and schooling to raised mirror the candidates skills. That is the resume: {resume}"""
),
expected_output= "Resume in markdown format that successfully highlights the candidate's {qualifications} and experiences",
# output_file="improved_resume.md",
context=[resume_feedback_task],
agent=resume_advisor
)
Now, the third agent ought to have the ability to recommend jobs based mostly on the {qualifications} and the popular job location of the candidate. For this, we’ll grant a software to our subsequent agent that allows it to go looking the web for job postings at a location.
We’ll use CrewAI’s SerperDevTool which integrates with Serper.dev for real-time internet search performance.
from crewai_tools import SerperDevTool
Now we import the API key from Serper. You’ll be able to generate your free Serper API key from https://serper.dev/api-key.
with open('/Customers/apoorv/Desktop/AV/Code/GAI/keys/serper.txt', 'r') as file:
serper_key = file.learn()
os.environ["SERPER_API_KEY"] = serper_key
search_tool = SerperDevTool()
Now we outline our ultimate agent which is the job_researcher agent. This agent will seek for jobs on the location based mostly on the resume improved by the resume_advisor agent. The construction of the agent is much like the above brokers with the one distinction being the addition of a brand new parameter, which is instruments. Instruments assist you to assign the required instruments to an agent which helps within the completion of the duty. Additionally, within the activity now we have added {location} in curly braces for dynamically altering it.
# Agent 3: Researcher
job_researcher = Agent(
function = "Senior Recruitment Marketing consultant",
purpose = "Discover the 5 most related, lately posted jobs based mostly on the improved resume recieved from resume advisor and the situation choice",
instruments = [search_tool],
verbose = True,
backstory = """As a senior recruitment advisor your prowess find essentially the most related jobs based mostly on the resume and site choice is unmatched.
You'll be able to scan the resume effectively, establish essentially the most appropriate job roles and seek for one of the best suited lately posted open job positions on the preffered location."""
)
research_task = Activity(
description = """Discover the 5 most related latest job postings based mostly on the resume recieved from resume advisor and site choice. That is the popular location: {location} .
Use the instruments to assemble related content material and shortlist the 5 most related, latest, job openings. Additionally present the hyperlinks to the job postings.""",
expected_output=(
"A bullet level checklist of the 5 job openings, with the suitable hyperlinks and detailed description about every job, in markdown format"
),
# output_file="relevant_jobs.md",
agent=job_researcher
)
Step 4: Creating the Crew and Reviewing the Output
Now we attain the ultimate step the place we bundle the brokers and the duties so as throughout the Crew performance of crewAI. It has 3 parameters:
- The brokers parameter lists the brokers within the order during which they are going to be known as and executed.
- The duties argument lists the duties outlined for every agent within the order of execution.
- And eventually, Verbose=True enables you to see the detailed output so you possibly can see what the brokers are doing.
Let’s run this.
crew = Crew(
brokers=[resume_feedback, resume_advisor, job_researcher],
duties=[resume_feedback_task, resume_advisor_task, research_task],
verbose=True
)
And we lastly launched our agentic system with the kickoff performance.
- crew.kickoff(inputs={…}): Begins the CrewAI execution, and takes in 2 inputs, resume and site.
- “resume”: res2: Helps you specify the resume for which the agentix system must work. In our case, we’re engaged on Anita’s resume.
- “location”: ‘New Delhi’: Specifies the place Anita is on the lookout for a job. And this will likely be New Delhi in our case.
result1 = crew.kickoff(inputs={"resume": res2, "location": 'New Delhi'})
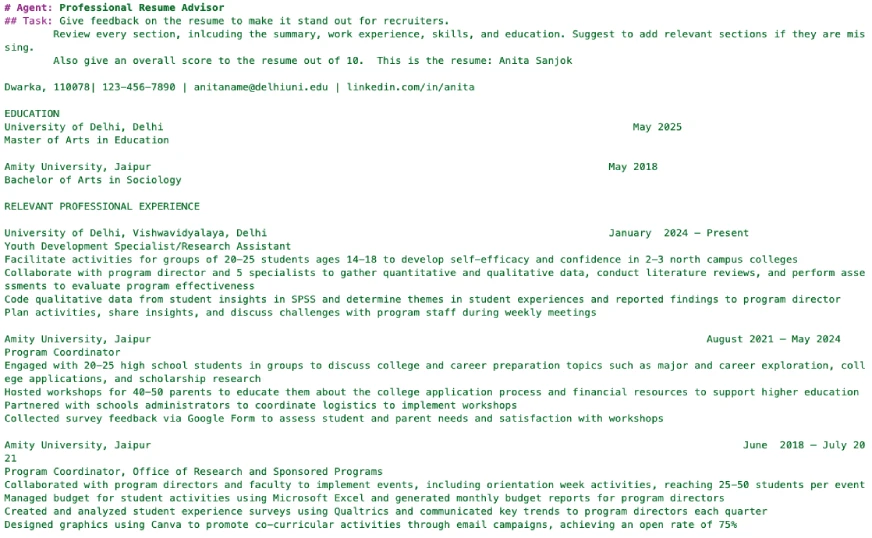
Looks as if our crew capabilities completely. Let’s print every agent’s output individually.
from IPython.show import Markdown, show
First, we’ll print resume_feedback agent’s output.
markdown_content = resume_feedback_task.output.uncooked.strip("```markdown").strip("```").strip()
# Show the Markdown content material
show(Markdown(markdown_content))
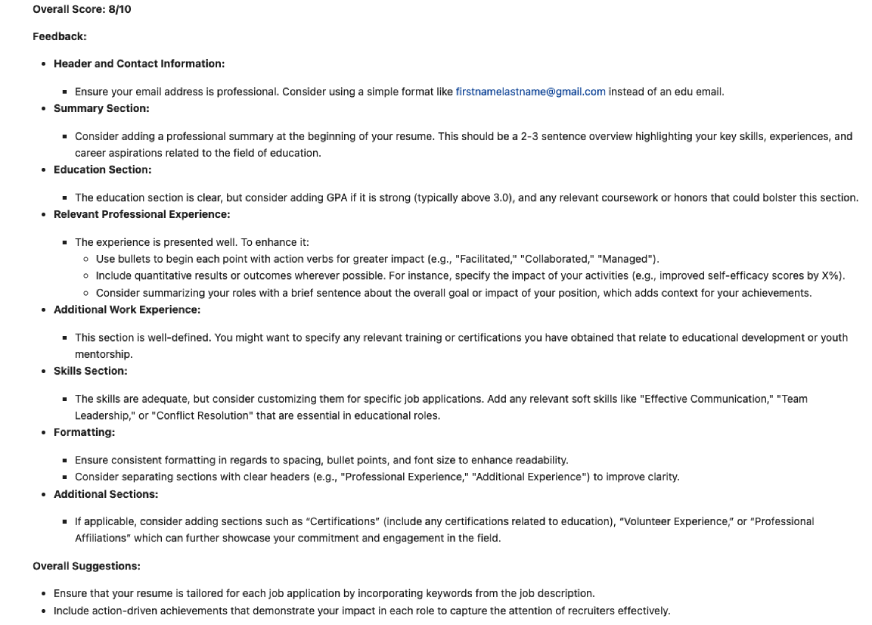
Then we print the resume_advisor agent’s output.
markdown_content = resume_advisor_task.output.uncooked.strip("```markdown").strip("```").strip()
# Show the Markdown content material
show(Markdown(markdown_content))
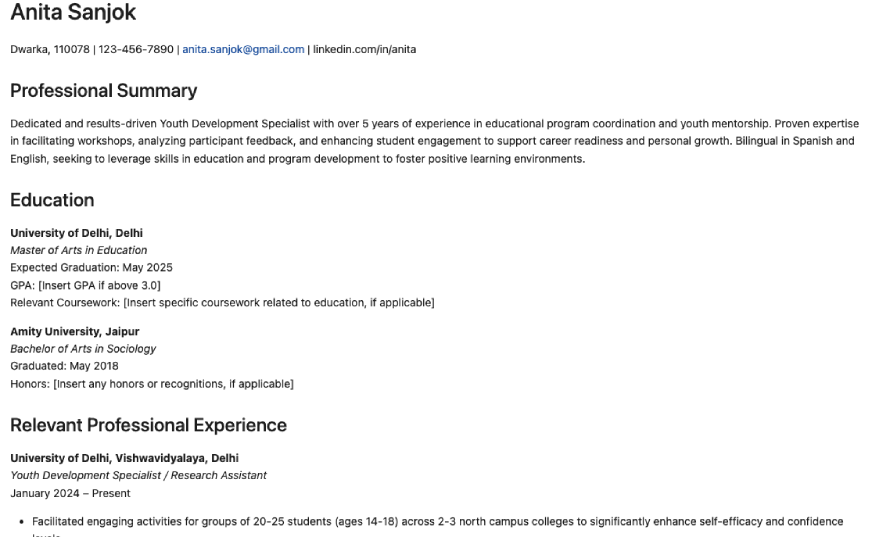
And eventually, we print the research_task agent’s output.
markdown_content = research_task.output.uncooked.strip("```markdown").strip("```").strip()
# Show the Markdown content material
show(Markdown(markdown_content))
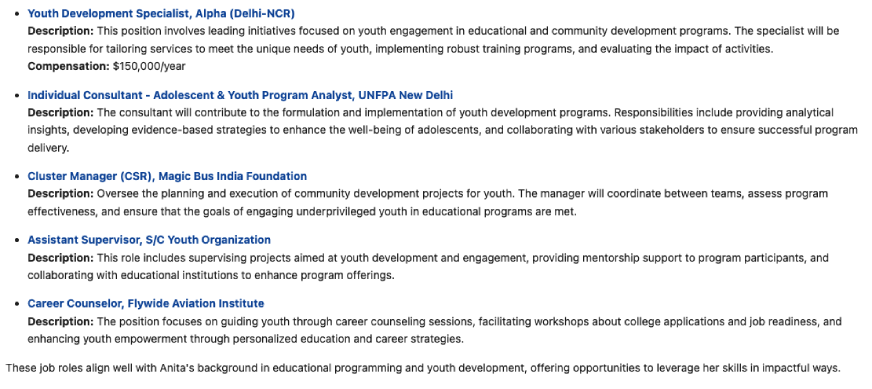
And there you’ve gotten it. Your totally purposeful resume evaluation agentic system with CrewAI.
Turning your CrewAI Resume Reviewer Agentic System right into a Internet App
One attention-grabbing factor we are able to do with our AI-driven resume optimization system is constructing a web-application for it.
Right here’s the interface of the app I constructed:
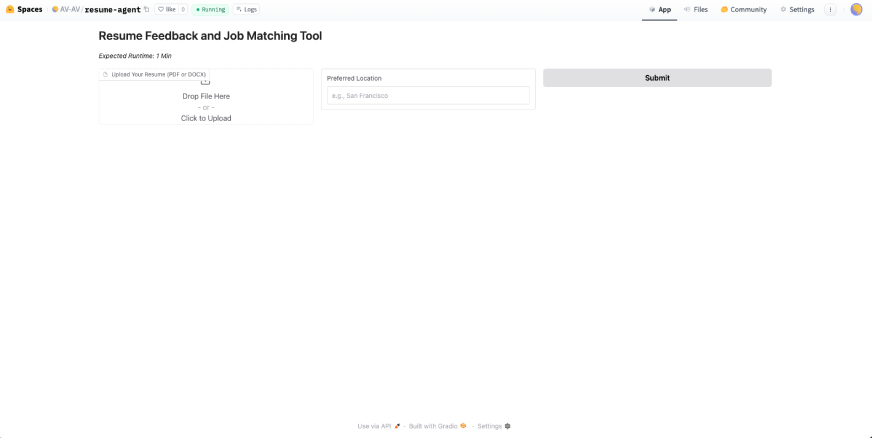
Now we have wrapped our code for resume evaluation agent with CrewAI in Gradio and hosted the ultimate product on Hugging Face Areas which has a liberal free tier.
Right here’s the way it works:
- First, drop your resume in a PDF or Phrase doc. The title of our candidate right here is Bruce Wayne.
- Subsequent, choose the popular job location for Bruce who’s our candidate. Let’s say San Francisco
- And hit Submit.
Since that is utilizing LLMs on the backend, GPT-4o-mini in our case, it is going to take a while to judge your resume, give a rating, and suggestions.
And as you possibly can see, Bruce received a rating of seven/10. And he has obtained in-depth section-wise suggestions from the CrewAI agentic system. Together with that he additionally received the revised resume and even solutions for jobs in San Francisco. How wonderful is that this!
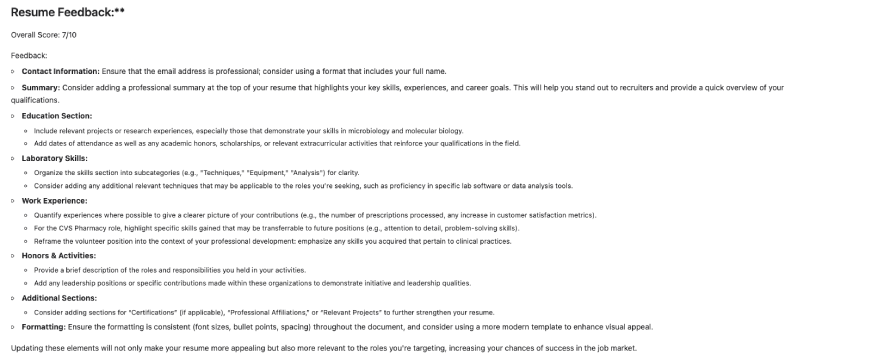
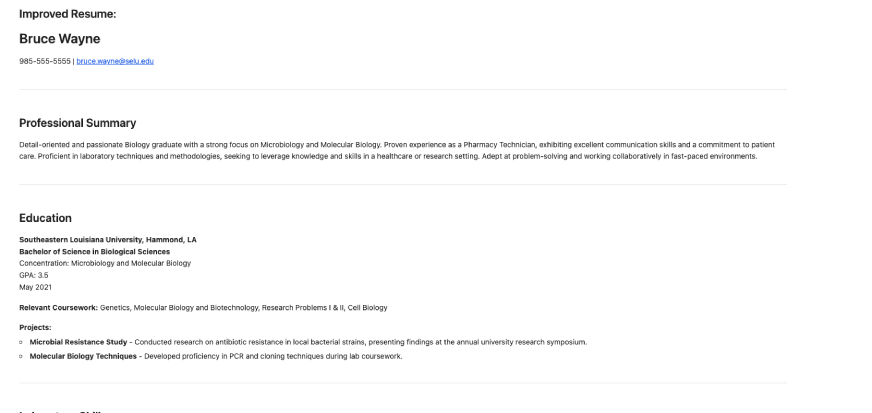
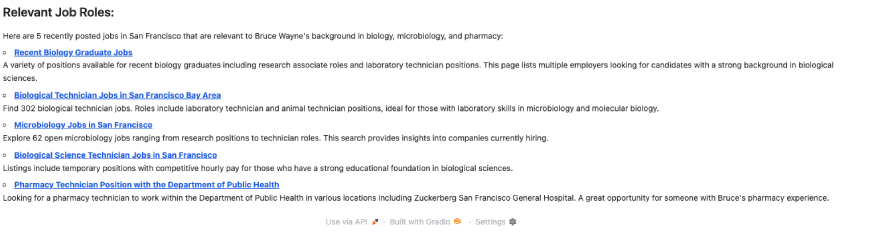
If you wish to replicate this CrewAI agentic system within the type of this web-application, you possibly can merely clone this repository or duplicate this area and alter the API key. Right here’s how that’s accomplished:
- First, click on on Settings after which click on on Clone repository within the dropdown.
- Then scroll right down to the variable and secrets and techniques setting.
- You’ll already discover the variables we created for the OpenAI key and Serper_Key. Merely click on on Exchange and add the API Key within the Worth. Then click on Save.
Additionally, you will get the code to construct this app from the CrewAI agentic system together with the Gradio code within the app.py file in information part of Hugging Face areas.

The press on app.py

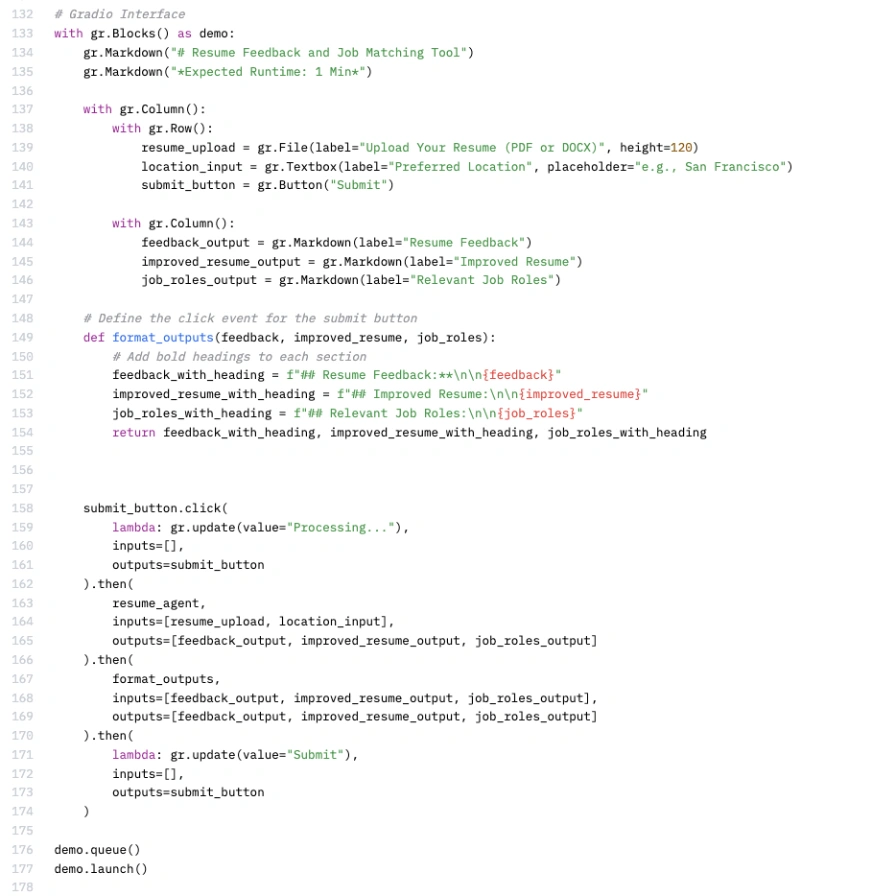
Doable Enhancements on the Resume Reviewer Agent
Like another agentic system, there’s plenty of scope for increasing the resume evaluation agent we’ve simply constructed with CrewAI. Listed here are a number of tricks to tinker round with:
- You’ll be able to construct a fourth agent that customizes the resume, based mostly on the job you choose.
- You’ll be able to construct one other agent ultimately to generate a canopy letter personalized for the job you wish to apply.
- It’s also possible to construct one other agent that can assist you to put together for the job you choose.
Conclusion
Constructing a resume reviewer agentic system is a game-changer for job seekers on the lookout for resume optimisation with AI-driven insights. This method effectively analyzes, refines, and suggests jobs based mostly on customized resume suggestions—all at a fraction of the price of premium resume optimizing providers. With CrewAI’s agentic capabilities, we’ve streamlined resume optimisation into an automatic but extremely efficient course of. However that is just the start! CrewAI is engaged on automating all types of duties and bringing much-needed effectivity to all industries. It’s certainly thrilling occasions forward!
Regularly Requested Questions
A. CrewAI is an open-source Python framework designed to help the event and administration of multi-agent AI programs. Utilizing CrewAI, you possibly can construct LLM-backed AI brokers that may autonomously make choices inside an surroundings based mostly on the variables current.
A. Sure! An AI agent just like the resume reviewer system we constructed utilizing CrewAI, may help optimize your resume. It might even advocate the suitable jobs for you based mostly in your {qualifications}, abilities, and site.
A. CrewAI can carry out numerous duties, together with reviewing resumes, writing resumes, trying to find jobs, making use of for jobs, getting ready cowl letters and extra.
A. In an effort to use this resume reviewer agent, the uploaded resume have to be in both PDF (.pdf) or Phrase (.docx) codecs.
A. Our resume reviewer agent makes use of OpenAI’s GPT-4o-mini for textual content processing and Serper.dev for real-time job search.
A. The job suggestions rely upon the standard of the resume and the AI’s skill to match abilities with job postings. Utilizing Serper.dev, the system retrieves essentially the most related and up to date job listings.
A. Fundamental Python programming abilities are required to arrange and modify the CrewAI-based system. Nonetheless, you possibly can clone a pre-built repository and replace the API keys to run it with out deep coding experience.